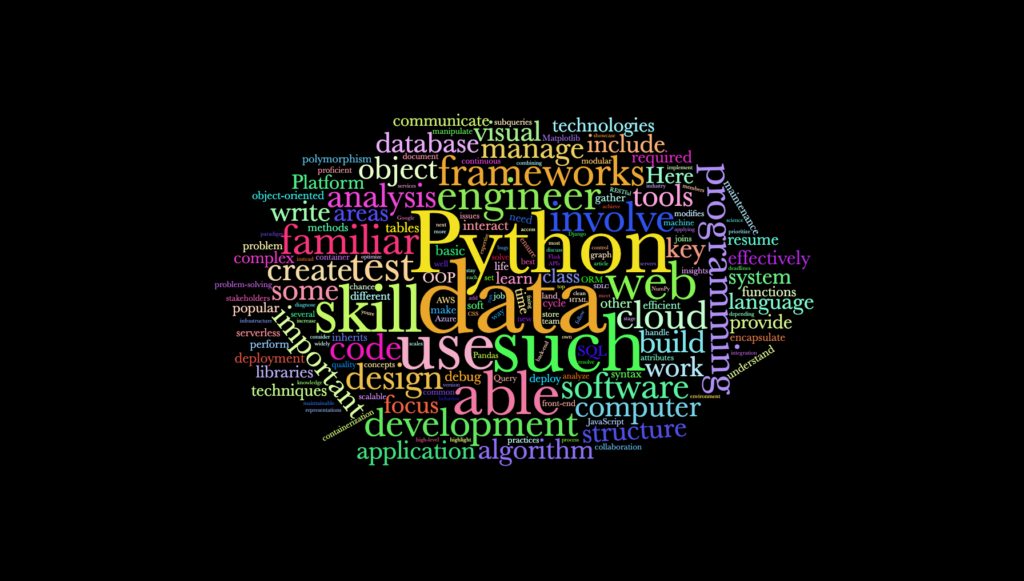
Understanding if __name__ == '__main__':
in Python: A Comprehensive Guide
If you’ve written or read Python code, you’ve probably come across this line:
if __name__ == '__main__':
It’s one of the most commonly seen — yet often misunderstood — lines in Python scripts. Despite its ubiquity, many Python beginners are unsure of what it does, why it’s used, and how it fits into the broader concepts of module execution and script execution.
In this guide, we’ll break down what if __name__ == '__main__':
means, why it’s important, and how to use it effectively. By the end, you’ll have a solid understanding of this key Python idiom and how it enhances code modularity and clarity.
Understanding Modules and Scripts in Python
What Is a Python Module?
A module is simply a Python file (.py
) that contains code — functions, classes, and variables — that you can import into other Python files.
# example_module.py
def greet():
print("Hello from the module!")
What Is a Python Script?
A script is a Python file that is run directly. When you run a script, Python executes the code line by line.
python example_module.py
Can a File Be Both a Module and a Script?
Yes! A Python file can be both:
- A module, when imported using
import
. - A script, when executed directly using the command line.
This duality is where if __name__ == '__main__':
becomes essential.
The __name__
Variable in Python
Python assigns a special built-in variable called __name__
to every module.
- If a file is run directly,
__name__
is set to'__main__'
. - If the file is imported as a module,
__name__
is set to the module’s name.
Example
# demo.py
print(f"__name__ is: {__name__}")
When run directly:
$ python demo.py
__name__ is: __main__
When imported:
import demo
# Output: __name__ is: demo
This behavior is the foundation for the if __name__ == '__main__':
check.
The Role of if __name__ == '__main__':
in Python
Why Use It?
This conditional block:
if __name__ == '__main__':
# Code to run
ensures that the code inside it only runs when the script is executed directly, not when it is imported.
Benefits
- Separation of concerns – Keeps test or demo code out of the module’s logic.
- Code reusability – Allows functions and classes to be reused without running unintended code.
- Organized entry point – Makes your script’s main execution flow clear.
Detailed Explanation with Code Examples
1. Simple Script Execution
# simple_script.py
def say_hello():
print("Hello!")
if __name__ == '__main__':
say_hello()
Output (when run directly):
Hello!
Behavior (when imported):
import simple_script
# No output
2. Importing a Module
# math_tools.py
def add(a, b):
return a + b
if __name__ == '__main__':
print(add(2, 3))
# main.py
import math_tools
print("Main script running")
Output:
Main script running
math_tools.py
doesn’t execute theprint(add(2, 3))
line.- Only the function is imported and ready for use.
3. Running Tests Within a Module
# calculator.py
def multiply(a, b):
return a * b
def test_multiply():
assert multiply(2, 3) == 6
assert multiply(-1, 5) == -5
print("All tests passed.")
if __name__ == '__main__':
test_multiply()
This lets developers run lightweight tests without needing an external testing framework.
4. Running Example Code
# greeter.py
def greet(name):
return f"Hello, {name}!"
if __name__ == '__main__':
print(greet("Alice"))
When used as a module:
import greeter
print(greeter.greet("Bob"))
5. Command-Line Interface (CLI)
# cli_tool.py
import sys
def echo_args():
print("Arguments:", sys.argv[1:])
if __name__ == '__main__':
echo_args()
Output:
$ python cli_tool.py hello world
Arguments: ['hello', 'world']
Use Cases and Best Practices
Common Use Cases
- Running tests.
- Demonstrating module usage.
- Creating command-line utilities.
- Preventing side-effects during import.
Best Practices
- Always place script-only logic inside
if __name__ == '__main__':
. - Keep modules clean and importable.
- Use this idiom as a script’s main entry point.
Potential Pitfalls
- Forgetting the check may result in code running unexpectedly on import.
- Overusing it in small scripts may add unnecessary complexity.
Advanced Considerations
Using with Command-Line Arguments
Combine if __name__ == '__main__':
with argparse
for sophisticated CLI tools:
import argparse
def main():
parser = argparse.ArgumentParser()
parser.add_argument('--name')
args = parser.parse_args()
print(f"Hello, {args.name}!")
if __name__ == '__main__':
main()
Integration with Testing Frameworks
Testing frameworks like pytest
ignore code in the __main__
block, keeping tests clean when importing.
Cross-Environment Compatibility
The behavior of __name__
is consistent across:
- IDLE
- Jupyter Notebooks
- Terminal
- IDEs like VS Code and PyCharm
Conclusion
The if __name__ == '__main__':
pattern is a fundamental part of Python programming. It provides a clean way to separate code meant for execution from code meant for reuse. Whether you’re building libraries, utilities, or simple scripts, understanding this idiom helps you write better organized and more modular Python code.
By mastering the difference between Python modules and scripts, and understanding how the __name__
variable works, you can write programs that are easier to test, maintain, and reuse.
Start applying this knowledge to your own projects and enjoy the benefits of cleaner, more Pythonic code.
Helpful Resources
- Official Python Documentation on
__name__
- PEP 8 – Python Style Guide
- Python Modules and Packages Tutorial – Real Python