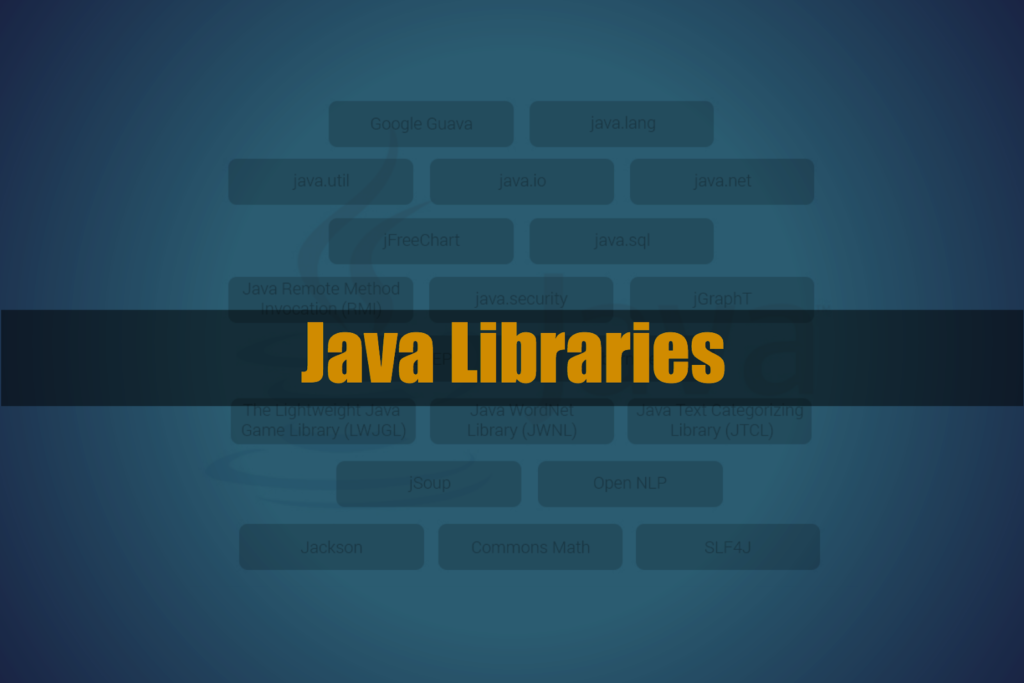
Free Open Source Java Chart Libraries: Best Picks for Developers
Data visualization is a crucial component of modern software development. Whether you’re building an analytics dashboard, tracking key performance indicators, or simply presenting data in an understandable format, charts and graphs are indispensable tools. For Java developers, leveraging the right chart library can save time, improve performance, and create visually appealing outputs.
In this article, we explore some of the best free and open-source Java chart libraries available. Each of these libraries offers unique features that cater to different requirements, making them ideal for a wide range of applications.
Why Use Open Source Chart Libraries?
Open-source libraries provide numerous advantages over proprietary alternatives, such as:
- Cost-Effectiveness: Free to use, reducing project costs.
- Flexibility: Full access to the source code for customization.
- Community Support: Active communities often provide quick fixes and enhancements.
- Transparency: No hidden functionalities; what you see is what you get.
Let’s dive into the best options for Java chart libraries that are free and open source.
1. JFreeChart
Overview
JFreeChart is one of the most popular and widely used open-source chart libraries for Java. It supports a wide variety of chart types and is suitable for both small projects and large-scale enterprise applications.
Key Features
- Supports various chart types, including bar, line, pie, scatter, and time series charts.
- Offers interactive features like zooming and tooltips.
- Can export charts to multiple formats, such as PNG, JPEG, PDF, and SVG.
- Works seamlessly with both Swing and JavaFX.
Use Case
Ideal for developers needing a robust charting library with extensive customization and export options.
Code Example
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.data.category.DefaultCategoryDataset;
import javax.swing.*;
public class JFreeChartExample {
public static void main(String[] args) {
DefaultCategoryDataset dataset = new DefaultCategoryDataset();
dataset.addValue(1, "Category 1", "January");
dataset.addValue(4, "Category 1", "February");
dataset.addValue(3, "Category 1", "March");
JFreeChart chart = ChartFactory.createBarChart(
"Monthly Sales",
"Month",
"Sales",
dataset
);
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new ChartPanel(chart));
frame.pack();
frame.setVisible(true);
}
}
2. XChart
Overview
XChart is a lightweight and easy-to-use library designed for creating simple yet attractive charts. Its minimalistic API makes it a favorite among developers looking for quick solutions.
Key Features
- Supports chart types like line, bar, scatter, pie, and more.
- Requires minimal boilerplate code.
- Offers a clean and modern appearance.
- Compatible with Swing and JavaFX.
Use Case
Perfect for developers who need straightforward and aesthetically pleasing charts with minimal effort.
Code Example
import org.knowm.xchart.XYChart;
import org.knowm.xchart.XYChartBuilder;
import org.knowm.xchart.SwingWrapper;
public class XChartExample {
public static void main(String[] args) {
double[] xData = new double[] {0.0, 1.0, 2.0, 3.0};
double[] yData = new double[] {2.0, 1.0, 0.0, 3.0};
XYChart chart = new XYChartBuilder()
.width(800)
.height(600)
.title("Simple Line Chart")
.xAxisTitle("X")
.yAxisTitle("Y")
.build();
chart.addSeries("Line", xData, yData);
new SwingWrapper<>(chart).displayChart();
}
}
3. Chart-FX
Overview
Chart-FX is designed specifically for JavaFX applications. It provides a powerful framework for creating high-performance and interactive charts.
Key Features
- Optimized for JavaFX environments.
- Offers dynamic updates for real-time data visualization.
- Includes advanced features like zooming, panning, and annotations.
- Provides support for 3D charts.
Use Case
Recommended for JavaFX developers working on applications requiring modern, interactive, and responsive charts.
Code Example
import de.gsi.chart.XYChart;
import de.gsi.chart.plugins.Zoomer;
import de.gsi.dataset.spi.DefaultDataSet;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class ChartFXExample extends Application {
@Override
public void start(Stage stage) {
XYChart chart = new XYChart("Dynamic Chart Example");
DefaultDataSet dataSet = new DefaultDataSet("Sample Data");
dataSet.set("X", new double[] {1, 2, 3, 4});
dataSet.set("Y", new double[] {4, 3, 2, 1});
chart.getDatasets().add(dataSet);
chart.getPlugins().add(new Zoomer());
stage.setScene(new Scene(chart, 800, 600));
stage.setTitle("ChartFX Example");
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
4. Orson Charts
Overview
Orson Charts is another Java charting library that excels in creating 3D charts. Developed by the creator of JFreeChart, it is well-suited for applications requiring advanced 3D visualizations.
Key Features
- Supports 3D bar, pie, and scatter charts.
- Renders charts with OpenGL for high performance.
- Includes support for JavaFX.
Use Case
Best for projects where 3D visualization is a key requirement.
Conclusion
Choosing the right chart library depends on your project’s requirements. Libraries like JFreeChart and XChart offer flexibility and simplicity for general use cases, while Chart-FX and Orson Charts cater to more specialized needs like real-time updates and 3D visualizations.
By leveraging these open-source options, you can create dynamic and visually stunning charts without incurring additional costs.