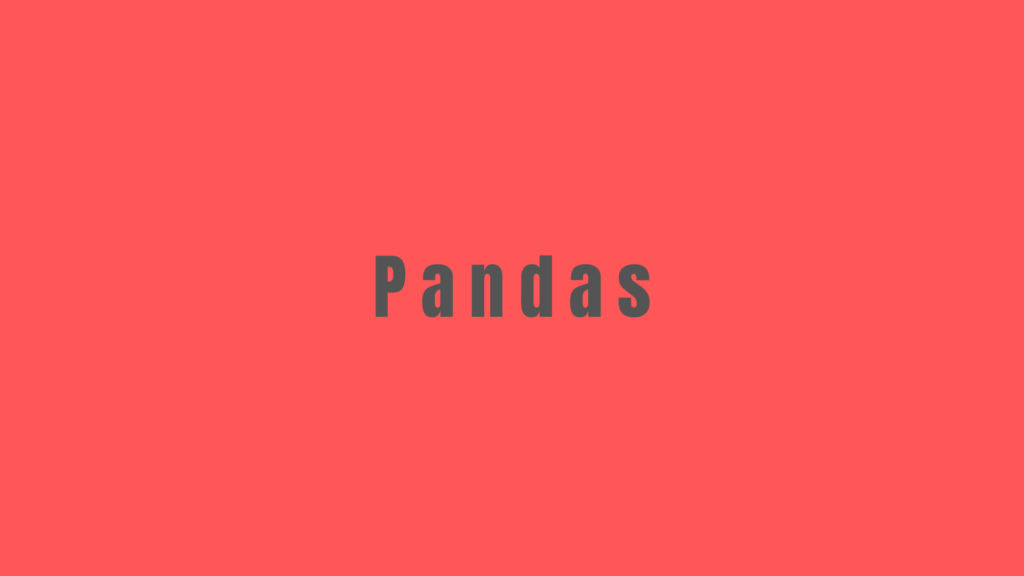
Declutter Your Data: The Ultimate Guide to Dropping NaN Values in Pandas
Handling missing data is one of the most common challenges in data analysis and manipulation. In Python, the Pandas library offers powerful tools to manage missing values seamlessly, enabling data scientists and analysts to maintain clean, reliable datasets.
In this guide, we’ll dive deep into how to drop NaN (Not a Number) values in Pandas, explore different use cases, and provide practical examples to help you master the process.
What Are NaN Values?
NaN stands for “Not a Number.” It is used to represent missing or undefined data in Pandas DataFrames and Series. Common reasons for NaN values include:
- Missing entries in datasets.
- Data type conversion errors.
- Issues during data scraping or file import.
NaN values can affect calculations, visualizations, and machine learning models, making it essential to address them effectively.
Importing Pandas
Before we dive into handling NaN values, let’s ensure Pandas is imported. Use the following command to import it:
pythonCopyEditimport pandas as pd
If you don’t have Pandas installed, install it via pip:
bashCopyEditpip install pandas
Identifying NaN Values in a Dataset
To drop NaN values, you first need to identify where they occur. Let’s look at a sample DataFrame:
pythonCopyEditimport pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie', None],
'Age': [25, 30, None, 22],
'City': ['New York', 'Los Angeles', None, 'Chicago']}
df = pd.DataFrame(data)
print(df)
Output:
sqlCopyEdit Name Age City
0 Alice 25.0 New York
1 Bob 30.0 Los Angeles
2 Charlie NaN None
3 None 22.0 Chicago
To identify NaN values, use the following Pandas functions:
isna()
orisnull()
: Returns a Boolean DataFrame indicating NaN values.notna()
ornotnull()
: Returns the opposite ofisna()
.
Example:
pythonCopyEditprint(df.isna())
Dropping NaN Values
The dropna()
function is the go-to method for removing NaN values in Pandas. Let’s explore how it works:
1. Dropping Rows with NaN Values
By default, dropna()
removes rows containing any NaN value:
pythonCopyEditcleaned_df = df.dropna()
print(cleaned_df)
Output:
sqlCopyEdit Name Age City
0 Alice 25.0 New York
1 Bob 30.0 Los Angeles
2. Dropping Columns with NaN Values
To drop columns with NaN values, set axis=1
:
pythonCopyEditcleaned_df = df.dropna(axis=1)
print(cleaned_df)
Output:
cssCopyEdit Name
0 Alice
1 Bob
2 Charlie
3 None
3. Controlling NaN Threshold
The thresh
parameter allows you to retain rows or columns with at least a certain number of non-NaN values:
pythonCopyEditcleaned_df = df.dropna(thresh=2)
print(cleaned_df)
Output:
sqlCopyEdit Name Age City
0 Alice 25.0 New York
1 Bob 30.0 Los Angeles
3 None 22.0 Chicago
4. Dropping NaN from Specific Columns
You can focus on specific columns by using the subset
parameter:
pythonCopyEditcleaned_df = df.dropna(subset=['Age'])
print(cleaned_df)
Output:
sqlCopyEdit Name Age City
0 Alice 25.0 New York
1 Bob 30.0 Los Angeles
3 None 22.0 Chicago
In-Place vs. Copy
By default, dropna()
returns a new DataFrame. If you want to modify the original DataFrame, set inplace=True
:
pythonCopyEditdf.dropna(inplace=True)
print(df)
Output:
sqlCopyEdit Name Age City
0 Alice 25.0 New York
1 Bob 30.0 Los Angeles
Use Cases and Best Practices
- Data Cleaning for Analysis: Dropping NaN values is helpful when missing data isn’t critical.
- Preparing Data for Machine Learning: While some models handle NaN values, most require a clean dataset.
- Exploratory Data Analysis (EDA): Dropping NaNs can simplify visualizations and summaries.
However, be cautious: dropping too many rows or columns might lead to information loss. Consider imputing missing values when appropriate.
Bonus: Filling NaN Values
Instead of dropping NaN values, you can fill them using the fillna()
function. For example:
pythonCopyEditdf['Age'].fillna(df['Age'].mean(), inplace=True)
print(df)
This replaces NaN values in the Age
column with the column’s mean value.
Conclusion
Handling NaN values in Pandas is a crucial skill for anyone working with data. The dropna()
function provides flexible options to clean your datasets efficiently. By understanding how and when to drop NaN values, you can ensure your data remains accurate, reliable, and ready for analysis.
Start experimenting with your datasets today, and watch how clean data can supercharge your insights!