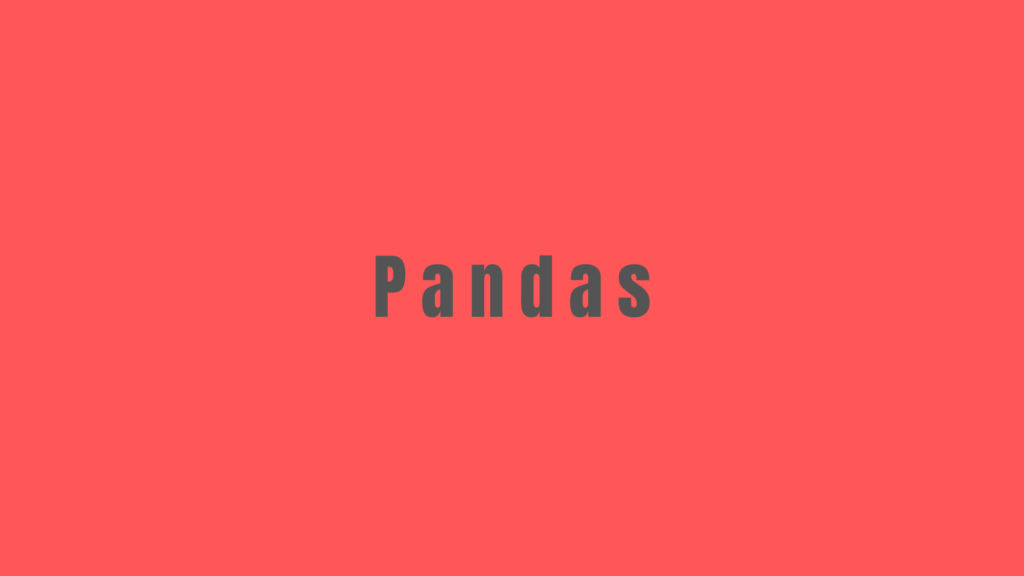
Pandas DataFrame Cleanup: Master the Art of Dropping Columns
Data cleaning and preprocessing are crucial steps in any data analysis project. When working with pandas DataFrames in Python, you’ll often encounter situations where you need to remove unnecessary columns to streamline your dataset. In this comprehensive guide, we’ll explore various methods to drop columns in pandas, complete with practical examples and best practices.
Understanding the Basics of Column Dropping
Before diving into the methods, let’s understand why we might need to drop columns:
- Remove irrelevant features that don’t contribute to analysis
- Eliminate duplicate or redundant information
- Clean up data before model training
- Reduce memory usage for large datasets
Method 1: Using drop() – The Most Common Approach
The drop()
method is the most straightforward way to remove columns from a DataFrame. Here’s how to use it:
pythonCopyimport pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({
'name': ['John', 'Alice', 'Bob'],
'age': [25, 30, 35],
'city': ['New York', 'London', 'Paris'],
'temp_col': [1, 2, 3]
})
# Drop a single column
df = df.drop('temp_col', axis=1)
# Drop multiple columns
df = df.drop(['city', 'age'], axis=1)
The axis=1
parameter indicates we’re dropping columns (not rows). Remember that drop()
returns a new DataFrame by default, so we need to reassign it or use inplace=True
.
Method 2: Using del Statement – The Quick Solution
For quick, permanent column removal, you can use Python’s del
statement:
pythonCopy# Delete a column using del
del df['temp_col']
Note that this method modifies the DataFrame directly and cannot be undone. Use it with caution!
Method 3: Drop Columns Using pop() – Remove and Return
The pop()
method removes a column and returns it, which can be useful when you want to store the removed column:
pythonCopy# Remove and store a column
removed_column = df.pop('temp_col')
Advanced Column Dropping Techniques
Dropping Multiple Columns with Pattern Matching
Sometimes you need to drop columns based on patterns in their names:
pythonCopy# Drop columns that start with 'temp_'
df = df.drop(columns=df.filter(regex='^temp_').columns)
# Drop columns that contain certain text
df = df.drop(columns=df.filter(like='unused').columns)
Conditional Column Dropping
You might want to drop columns based on certain conditions:
pythonCopy# Drop columns with more than 50% missing values
threshold = len(df) * 0.5
df = df.dropna(axis=1, thresh=threshold)
# Drop columns of specific data types
df = df.select_dtypes(exclude=['object'])
Best Practices for Dropping Columns
- Make a Copy First pythonCopy
df_clean = df.copy() df_clean = df_clean.drop('column_name', axis=1)
- Use Column Lists for Multiple Drops pythonCopy
columns_to_drop = ['col1', 'col2', 'col3'] df = df.drop(columns=columns_to_drop)
- Error Handling pythonCopy
try: df = df.drop('non_existent_column', axis=1) except KeyError: print("Column not found in DataFrame")
Performance Considerations
When working with large datasets, consider these performance tips:
- Use
inplace=True
to avoid creating copies: pythonCopydf.drop('column_name', axis=1, inplace=True)
- Drop multiple columns at once rather than one by one: pythonCopy
# More efficient df.drop(['col1', 'col2', 'col3'], axis=1, inplace=True) # Less efficient df.drop('col1', axis=1, inplace=True) df.drop('col2', axis=1, inplace=True) df.drop('col3', axis=1, inplace=True)
Common Pitfalls and Solutions
- Dropping Non-existent Columns pythonCopy
# Use errors='ignore' to skip non-existent columns df = df.drop('missing_column', axis=1, errors='ignore')
- Chain Operations Safely pythonCopy
# Use method chaining carefully df = (df.drop('col1', axis=1) .drop('col2', axis=1) .reset_index(drop=True))
Real-World Applications
Let’s look at a practical example of cleaning a dataset:
pythonCopy# Load a messy dataset
df = pd.read_csv('raw_data.csv')
# Clean up the DataFrame
df_clean = (df.drop(columns=['unnamed_column', 'duplicate_info']) # Remove unnecessary columns
.drop(columns=df.filter(regex='^temp_').columns) # Remove temporary columns
.drop(columns=df.columns[df.isna().sum() > len(df)*0.5]) # Remove columns with >50% missing values
)
Integration with Data Science Workflows
When preparing data for machine learning:
pythonCopy# Drop target variable from features
X = df.drop('target_variable', axis=1)
y = df['target_variable']
# Drop non-numeric columns for certain algorithms
X = X.select_dtypes(include=['float64', 'int64'])
Conclusion
Mastering column dropping in pandas is essential for effective data preprocessing. Whether you’re using the simple drop()
method or implementing more complex pattern-based dropping, understanding these techniques will make your data cleaning process more efficient and reliable.
Remember to always consider your specific use case when choosing a method, and don’t forget to make backups of important data before making permanent changes to your DataFrame.
Now you’re equipped with all the knowledge needed to effectively manage columns in your pandas DataFrames. Happy data cleaning!