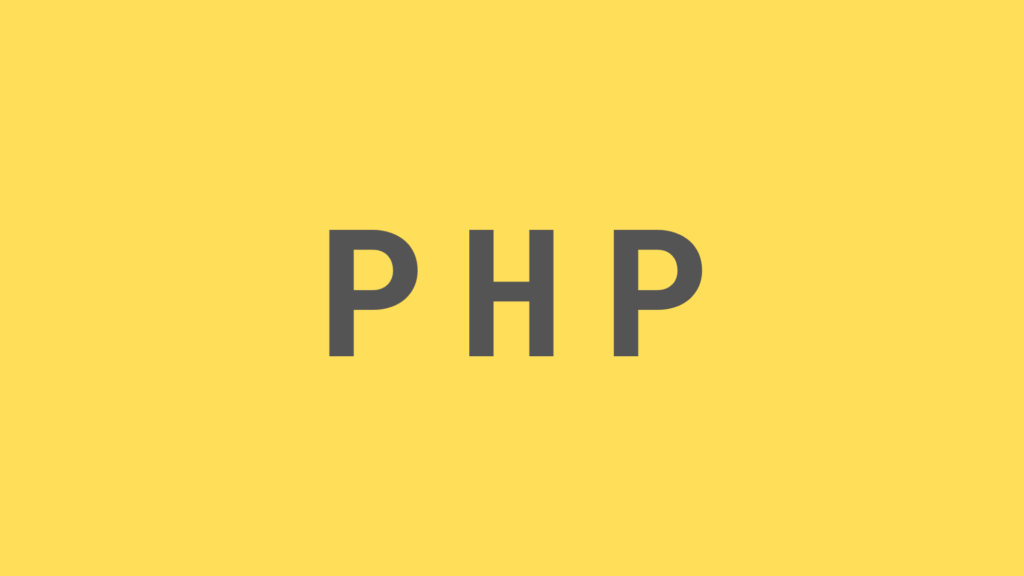
Supercharge Your WordPress Development with These 10 PHP Snippets
WordPress powers over 40% of the web, making it one of the most popular platforms for developers and bloggers alike. Whether you’re customizing themes, building plugins, or tweaking functionality, having a handy set of PHP code snippets can save you hours of work. Here are 10 practical PHP snippets every WordPress developer should keep in their toolkit.
1. Disable WordPress Admin Bar for Non-Admins
The admin bar can clutter the frontend for non-admin users. Use this snippet to disable it for everyone except administrators:
add_action('after_setup_theme', function() {
if (!current_user_can('administrator')) {
show_admin_bar(false);
}
});
This ensures a clean frontend experience for subscribers and other roles.
2. Change Default Login Logo URL
Customizing the WordPress login page is a common requirement. Use this snippet to change the logo URL on the login screen:
add_filter('login_headerurl', function() {
return home_url(); // Redirect to your website's homepage
});
Combine this with CSS to fully brand the login page for clients or personal projects.
3. Redirect Users After Login Based on Role
Direct users to specific pages after login based on their roles:
add_filter('login_redirect', function($redirect_to, $request, $user) {
if (isset($user->roles) && is_array($user->roles)) {
if (in_array('administrator', $user->roles)) {
return admin_url();
} elseif (in_array('editor', $user->roles)) {
return home_url('/editor-dashboard');
} else {
return home_url('/welcome');
}
}
return $redirect_to;
}, 10, 3);
This snippet improves user navigation and role-specific workflows.
4. Custom Excerpt Length
Control the excerpt length in posts to maintain a consistent look across your site:
add_filter('excerpt_length', function($length) {
return 20; // Set the excerpt length to 20 words
});
Pair this with a custom read more
link for better UX:
add_filter('excerpt_more', function() {
return '... <a href="' . get_permalink() . '">Read More</a>';
});
5. Remove WordPress Version Number
Hiding the WordPress version number improves security by making it harder for attackers to target vulnerabilities:
remove_action('wp_head', 'wp_generator');
This simple snippet removes the version meta tag from the HTML source.
6. Add Custom Image Sizes
Define and use custom image sizes for different parts of your theme:
add_action('after_setup_theme', function() {
add_image_size('custom-thumb', 400, 300, true); // Cropped image size
});
To use this image size in your theme:
echo wp_get_attachment_image($attachment_id, 'custom-thumb');
7. Disable WordPress Auto Updates
Sometimes you want to disable automatic updates to maintain more control:
add_filter('automatic_updater_disabled', '__return_true');
You can also disable specific updates, such as plugin updates:
add_filter('auto_update_plugin', '__return_false');
8. Custom Maintenance Mode
Display a maintenance mode page while working on your site:
add_action('template_redirect', function() {
if (!current_user_can('administrator') && !is_user_logged_in()) {
wp_die(
'<h1>Under Maintenance</h1><p>We’ll be back shortly!</p>',
'Maintenance Mode',
array('response' => 503)
);
}
});
This ensures only admins can access the site during maintenance.
9. Limit Post Revisions
Restrict the number of post revisions saved in the database to optimize performance:
define('WP_POST_REVISIONS', 5); // Limit to 5 revisions per post
Add this line to your wp-config.php
file to apply globally.
10. Custom Dashboard Widget
Add a custom widget to the WordPress admin dashboard for quick links or messages:
add_action('wp_dashboard_setup', function() {
wp_add_dashboard_widget(
'custom_dashboard_widget',
'Welcome to Your Dashboard',
function() {
echo '<p>Need help? Visit our <a href="https://example.com/support">support center</a>.</p>';
}
);
});
This snippet is great for client sites where you can provide tailored guidance.
Wrapping Up
These 10 PHP snippets cover some of the most common tasks WordPress developers encounter. By incorporating these into your workflow, you’ll save time and enhance the functionality of your projects. Bookmark this cheat sheet and customize these snippets to suit your specific needs!