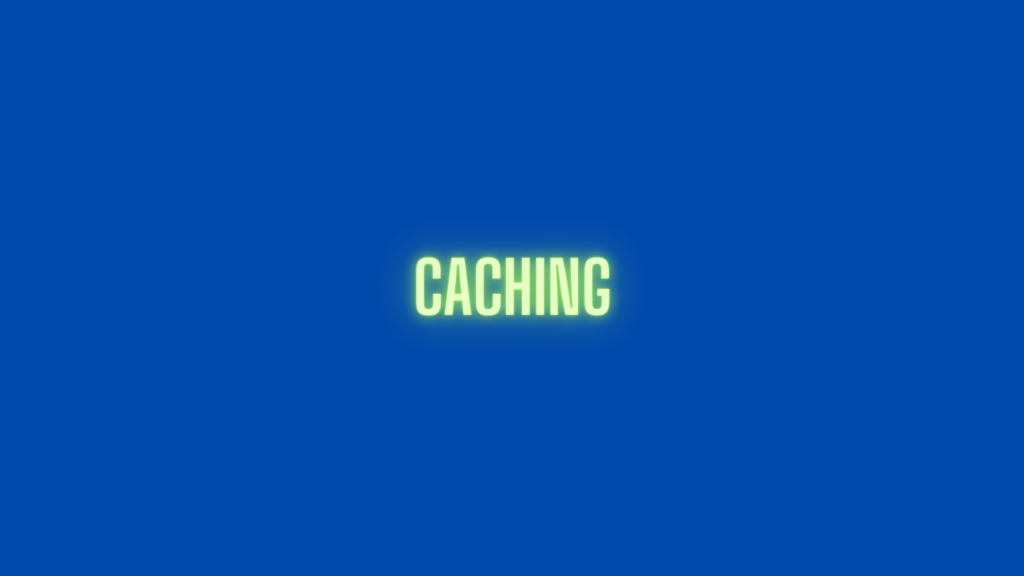
Best In-Memory Caching Frameworks in Node.js
When building high-performance applications with Node.js, efficient data retrieval and response times are crucial. In-memory caching frameworks provide a way to store and quickly access frequently used data, reducing the load on databases and APIs. This guide explores the best in-memory caching frameworks to elevate your Node.js applications.
What is In-Memory Caching?
In-memory caching temporarily stores data in memory (RAM) instead of a persistent data store like a database. This approach significantly reduces latency because accessing RAM is faster than querying a database. Common use cases include caching API responses, session data, and computational results.
Why Use Caching in Node.js?
- Improved Performance: Reduces response time for frequently accessed data.
- Reduced Costs: Lowers database and server workload.
- Scalability: Helps applications handle higher traffic without degradation.
Top In-Memory Caching Frameworks for Node.js
1. Redis
Redis is a widely-used in-memory data structure store supporting strings, hashes, lists, and more.
- Features:
- Persistent caching with disk snapshots.
- Built-in support for pub/sub messaging.
- Advanced data structures for versatile use cases.
- Why Choose Redis:
Redis integrates seamlessly with Node.js using libraries likeioredis
ornode-redis
. It’s ideal for caching, session storage, and real-time analytics.
2. Memcached
Memcached is a high-performance, distributed caching system.
- Features:
- Simple key-value storage.
- Extremely lightweight and fast.
- Why Choose Memcached:
Perfect for applications needing basic key-value caching without complex features. Thememcached
Node.js library simplifies integration.
3. Node-Cache
Node-Cache is a lightweight and simple caching solution designed for Node.js applications.
- Features:
- Pure JavaScript implementation.
- Support for TTL (time-to-live) expiration.
- Why Choose Node-Cache:
Ideal for small-scale applications needing an easy-to-implement caching mechanism.
4. Apache Ignite
Apache Ignite combines caching with distributed computing capabilities.
- Features:
- In-memory data grid.
- Built-in machine learning and SQL support.
- Why Choose Apache Ignite:
Suitable for applications requiring caching and distributed computation in one framework.
5. Hazelcast
Hazelcast is a distributed in-memory data grid and caching solution.
- Features:
- Clustered caching for fault tolerance.
- Advanced features like map-reduce and event-driven computation.
- Why Choose Hazelcast:
A great option for Node.js applications with heavy distributed processing needs.
How to Choose the Right Framework
- Project Size: For small projects, Node-Cache or Memcached may suffice. For larger, scalable apps, Redis or Hazelcast is better.
- Complexity of Data: If your application requires advanced data structures, Redis is an excellent choice.
- Real-Time Features: Applications needing real-time data streaming might benefit from Redis or Hazelcast.
Best Practices for Using In-Memory Caching
- Set Expiry Times: Use TTL to automatically expire outdated data.
- Cache Only Necessary Data: Avoid caching everything; focus on frequently accessed or computationally expensive data.
- Monitor Cache Performance: Regularly monitor hit/miss ratios to optimize cache effectiveness.
Conclusion
In-memory caching frameworks are essential for building efficient and scalable Node.js applications. Whether you choose Redis for its advanced features or Node-Cache for simplicity, implementing caching can drastically enhance your app’s performance.
Start caching today and see the difference it makes in your Node.js projects!