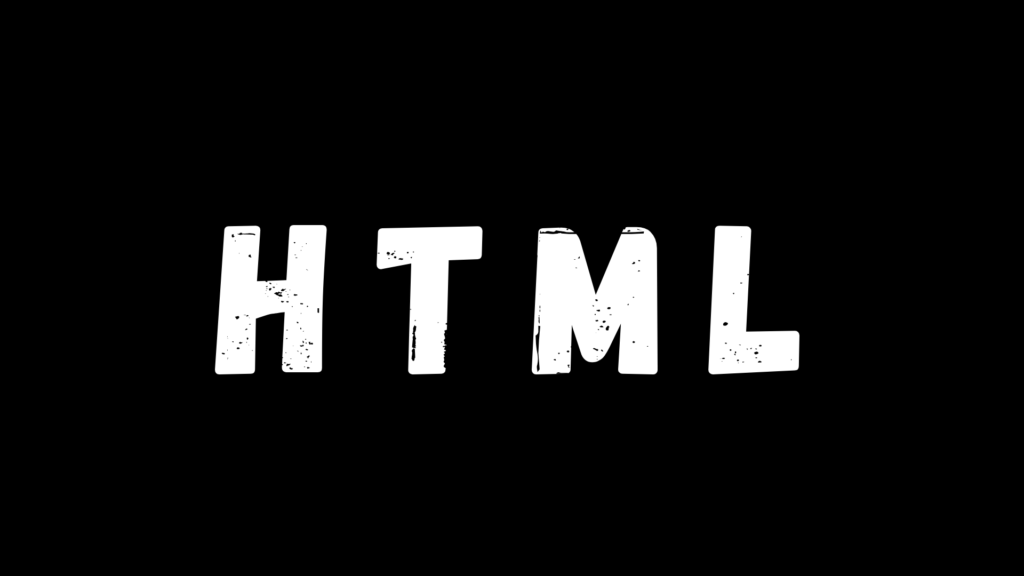
HTML Box Tutorial: Learn to Create and Style Boxes
Creating a box in HTML is one of the first things you’ll learn when diving into web development. Boxes are fundamental building blocks for structuring and styling content on a webpage. Whether you’re looking to add emphasis to text, create a container for an image, or style a layout, mastering HTML boxes is essential. In this guide, we’ll walk you through the steps to create and customize a box using HTML and CSS. Let’s get started!
What is an HTML Box?
An HTML box is essentially any element on a webpage that can hold content. It could be a “div”, “section”, or even a “span”, styled to look like a box. By combining HTML for structure and CSS for styling, you can create visually appealing boxes for any purpose.
Step 1: Setting Up Your HTML File
Before creating a box, you’ll need a basic HTML file to work with. If you don’t already have one, here’s how to set it up:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML Box Example</title>
<style>
/* Add your CSS here */
</style>
</head>
<body>
<!-- Add your content here -->
</body>
</html>
This structure sets the foundation for your webpage. The “” section in the “” is where you’ll add CSS to style your box.
Step 2: Adding an HTML Element
Next, add an HTML element to serve as your box. A common choice is the “” element because it’s a versatile container:
<div class="box">
This is my box!
</div>
The “class” attribute allows you to target this specific element in your CSS for styling.
Step 3: Styling the Box with CSS
Now comes the fun part—styling your box! Use the “” section or an external CSS file to add some personality to your box. Here’s an example:
<style>
.box {
width: 200px; /* Width of the box */
height: 100px; /* Height of the box */
background-color: lightblue; /* Background color */
color: white; /* Text color */
border: 2px solid navy; /* Border around the box */
border-radius: 10px; /* Rounded corners */
text-align: center; /* Center the text horizontally */
line-height: 100px; /* Center the text vertically */
margin: 20px auto; /* Center the box on the page */
box-shadow: 5px 5px 15px rgba(0, 0, 0, 0.3); /* Add a shadow */
}
</style>
This CSS creates a stylish, centered box with a shadow and rounded corners. You can customize the styles to match your preferences.
Step 4: Experimenting with Advanced Features
Once you’ve mastered the basics, try enhancing your box with additional CSS properties. Here are a few ideas:
- Add a Gradient Background:
background: linear-gradient(to right, lightblue, navy);
- Animate the Box:
.box {
transition: transform 0.3s;
}
.box:hover {
transform: scale(1.1); /* Make the box larger on hover */
}
- Use Flexbox for Layout:
.display-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Full viewport height */
}
.box {
flex-basis: 200px;
}
Step 5: Testing and Refining
Once you’ve created and styled your box, test it in different browsers to ensure it looks good everywhere. Modern browsers like Chrome, Firefox, and Edge support most CSS properties, but it’s always good to double-check.
Common Pitfalls to Avoid
- Forgetting to Include CSS: Without CSS, your box will just be plain text or content.
- Missing the Closing Tags: Ensure every HTML tag is properly closed.
- Overcomplicating Styles: Start simple and build complexity gradually.
Conclusion
Creating a box in HTML is an exciting first step in web development. With just a few lines of code, you can design functional and attractive elements for your website. As you experiment and practice, you’ll discover endless possibilities for customizing and enhancing your boxes. So grab your code editor and start creating!