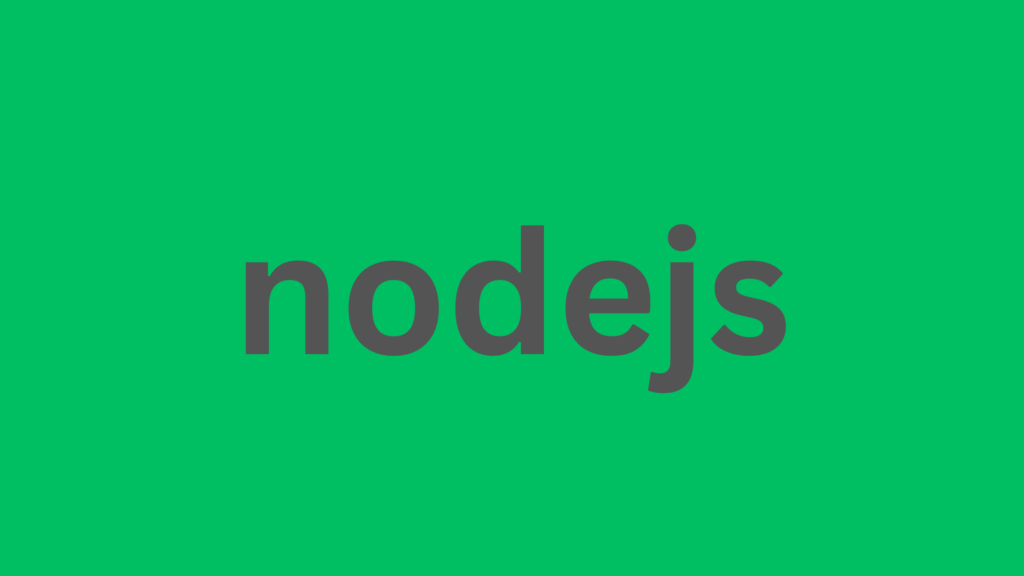
Understanding Console.log in Node.js: How It Works and Where It’s Stored
In the world of JavaScript, especially within the Node.js runtime, developers rely heavily on console.log()
for debugging and tracking the flow of their applications. But have you ever wondered where all those console.log()
statements actually go? While it might seem straightforward in the browser, things work a bit differently in Node.js, and understanding this can help you manage logs effectively and debug efficiently.
In this article, we’ll dive into the workings of console.log()
in Node.js, where its output goes, and how to handle logs in various environments.
Understanding console.log()
in Node.js
At its core, console.log()
in Node.js functions similarly to how it does in a browser environment. It prints out text or values to a destination that allows you to inspect them. However, unlike in browsers where console.log()
outputs directly to the web console, in Node.js, the logs are sent to different streams based on the environment the code is running in.
Node.js processes operate in a server-side environment, and as such, the behavior of console.log()
is tied to standard I/O (Input/Output) streams. These streams include:
- stdout (Standard Output): This is where most of the output generated by
console.log()
goes. By default, anything you print withconsole.log()
in Node.js is sent tostdout
, which typically means it shows up in the terminal or command line where the Node.js process is running. - stderr (Standard Error): This is where error messages and warning information are sent. Functions like
console.error()
use this stream to output error-related logs. Although errors and normal logs might look the same in the terminal, they are actually handled by different streams.
The Role of Streams: stdout vs. stderr
Understanding the distinction between stdout
and stderr
is essential for log management. The separation allows developers to better manage logs, especially when dealing with automated processes or production environments where logs are filtered or directed to specific logging systems.
- stdout (used by
console.log()
andconsole.info()
): Outputs standard application logs, information messages, or debugging content. - stderr (used by
console.error()
andconsole.warn()
): Outputs error logs or warnings, helping separate critical issues from regular information.
In many server environments, logs from both stdout
and stderr
may be redirected to files, external logging systems, or error monitoring tools.
Where Does console.log()
Output Go?
In most cases, console.log()
outputs to the terminal or console from which the Node.js application is running. Here’s how it works depending on your environment:
- Local Development: When you’re running a Node.js application on your local machine,
console.log()
typically outputs directly to the terminal window (or command prompt) where you started the application. This is helpful for debugging as you can see the output immediately. - Production Environments: In a production server environment, things are a bit different. Often, Node.js applications are started using process managers like PM2 or forever, or they are run inside containers such as Docker. In these environments, the logs from
console.log()
are usually directed to log files or centralized logging systems like Winston or Logstash. This allows for better log management, historical record-keeping, and easier debugging when things go wrong. - Cloud Platforms: If your Node.js application is deployed on a cloud platform like AWS, Google Cloud, or Heroku, the
console.log()
output can be directed to cloud-based logging solutions like CloudWatch Logs (AWS) or Google Cloud Logging. These platforms store your logs and allow you to search through them, set up alerts, and integrate logs into monitoring systems.
Managing and Redirecting Logs
In modern Node.js applications, managing logs effectively goes beyond just using console.log()
. As your application grows and scales, a logging strategy is crucial. Here are a few common ways to manage and redirect logs in Node.js:
- Using Logging Libraries: Instead of relying solely on
console.log()
, developers often use logging libraries like Winston or Bunyan. These libraries offer advanced features such as:- Log levels (debug, info, warn, error)
- Log rotation (to prevent log files from growing too large)
- Structured logging (to make logs easier to parse and analyze)
- Support for logging to multiple destinations (files, databases, external services)
- Redirecting stdout and stderr: If you need more control over where your logs are stored, you can redirect the
stdout
andstderr
streams to files or other destinations. Here’s a basic example
const fs = require('fs');
const stdout = fs.createWriteStream('stdout.log');
const stderr = fs.createWriteStream('stderr.log');
console.log = function (msg) { stdout.write(msg + '\n'); };
console.error = function (msg) { stderr.write(msg + '\n'); };
- Log Monitoring and Alerts: In production, it’s common to set up log monitoring systems. Tools like Datadog, Sentry, and Elastic Stack can automatically monitor logs for errors, performance issues, or specific events. You can set up alerts that trigger when certain conditions are met (e.g., a specific error appears repeatedly), helping you stay on top of issues in real time.
Conclusion
In Node.js, console.log()
may seem like a simple tool, but where those logs go and how they are managed is a crucial aspect of application development, especially as your projects grow in complexity. Whether you’re running a local server or a large-scale cloud-based app, understanding the destination of your console.log()
outputs helps you debug faster, manage logs better, and keep your applications running smoothly.
By implementing good logging practices, using the right tools, and being mindful of where your logs go, you can ensure your Node.js applications are robust, well-monitored, and easy to maintain.