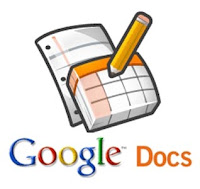
Google form auto reply email is not a in built feature, however we can achieve it by simple script. There is a simple way to Send the contact form in the form of email to your inbox described here. However, if you want a automated reply email sent to your customers it needs to be done using some JavaScript function which can be easily configured to be called inside Google forms.
Google form automatic email can be easily triggered on the form submission.
Below are the steps to configure a automated response email for your Google form.
Why Send Auto Reply
The default Google contact form feature provides a google docs spreadsheet with user responses, and responding to them needs to be done using manual emails. Sometimes its not feasible to reply back to people immediately, I have found some of the main reasons as listed below
- In case your site is really popular and lot of people are trying to reach you.
- There may be lot of spam contact messages.
- There may be worldwide customers trying to reach you during odd working hours/weekends.
- You may be on vacation.
- You need some time to do research before you respond.
- Some of the categories need not be attended since your answers can be canned. e.g. you want to thank your customer for providing feedback or some help.
Below are simple steps to setup the auto reply email.
- Create Contact Form
- Add JavaScript Function for Auto Reply Email
- Google forms auto response will be sent immediately with email content configured in your script. Make sure to update the email response “message” in the script before you start using this.
- Field names are case sensitive. Please make sure the field names are exactly matching in the script. (e.g. Email, Subject)
- Make sure to update the script with your own email address on this line
var myemail = “abc@youmail.com”;
- In case you want the auto reply content to be really big make sure you split it into multiple lines (and use Javasctipt append) like below instead of keeping all text in one double quote.
var message = “Thanks for contacting FromDev.com. We will get in touch with you shortly. nn—–nn”
+ “Another line n”
+ “Another line”; - Add a Trigger
- Tools – Script Editor – Resources – Current Script’s Triggers
- Select Function name “sendAutoReply”
- Select “From Spreadsheet”
- Select “On Form Submit”
- Save
- Authorize Script
- Lets Test our Script
- Something went wrong?
In case you have not created the contact form before, don’t worry. Its really easy and free. Checkout this simple Youtube Video about “How To Create Contact Form on Google Docs“. This example assumes you have at least four fields in your contact form (Name, Email, Subject and content/message). Checkout our contact form for details.
Below is the JavaScript code which can be used to send automated email reply. This code uses Google MailApp utility to send reply. Follow these steps and copy the below code Tools – Script Editor – Copy Below code into the text editor. – Save.
function sendAutoReply(e) { // Remember to replace abc@youmail.com with your own email address // This will be used to send you a email notification in case of error on form // for example if someone entered a wrong email address. var myemail = Session.getActiveUser().getEmail(); //Leave this field blank - it will be auto populated from "Email" field in // your contact form. Make sure you have a "Email" Field in your contact form. var email = ""; // This is going to be the subject of reply email // You can change it as per your requirements var subject = "Re: "; // The variable e holds all the form values in an array. // Loop through the array and append values to the body. var message = "Thanks for contacting FromDev.com. We will get in touch with you shortly. nn-----nn"; var columns, mysheet; try { mysheet = SpreadsheetApp.getActiveSheet(); columns = mysheet.getRange(1, 1, 1, mysheet.getLastColumn()).getValues()[0]; if (e) { // This is the submitter's email address var em = e.namedValues["Email"]; if(em) { email = em.toString(); } else { throw {'message':'Email Field is required. Please add a field called Email in your sheet.'} } var sub = e.namedValues["Subject"]; if(sub) { subject += sub.toString(); } // Only include form values that are not blank for (var keys in columns) { var key = columns[keys]; if (e.namedValues[key]) { message += key + ' :: ' + e.namedValues[key] + "nn"; } } // We are using JavaScript MailApp util of Google Apps Script // that sends the email. MailApp.sendEmail(email, subject, message, { replyTo: myemail }); } else { var err = 'Required Input params are passed only during live form submission. Please try the script by submitting the live form.'; Logger.log(err); throw { 'message': err }; } } catch (e) { MailApp.sendEmail(myemail, "Error in Auto replying to contact form submission. No reply was sent.", e.message + ' nncolumns: ' + columns + ' nnmessage: ' + message); } }
Special Caution
Please make sure you take care of following things when you create a form and update any code in script.
The next steps is to add a trigger to run the script on form submission. The trigger is a simple configuration to tell google docs to listen to specific event and take action on it. We are simply going to create a trigger at the event of form submission and execute our auto reply email script in the action response to that event. Follow these steps
You need to Authorize Script to be run by Google API. The authorization step is really important since Google API will not run the script unless its authorized by the creator. Therefore make sure you are creating the script using the same account which owns the contact form.
I believe in test driven development, and as always try to make sure your setup is working fine. This is really simple, just do the form submission using a email address and see if the auto reply email is received as required.
Your script is still not working? There can be following reasons – Please make sure you have the exact same field names in your contact form. (Specially for Email and Subject). Checkout our contact form. – If someone enters a invalid email address or other error happens on your form, you would be notified with a email similar to below snapshot.
March 2013: Updated: Error Handling and Reply to Option as per reader feedback.
Hope you found this tutorial useful. Which contact form application are you using?
Nice! Thank you, it is very useful.
Jirka – Thanks for your comment. I am glad to know that it was helpful.
This comment has been removed by the author.
Hi,
I'm in a bit of a picle.
Is there a way that the email that you receive has the email address of the user in the from field, so that when you hit reply it, it replies to the user and not the Gmail address?
Thanks
I have not tried this however there is a way to set ReplyTo option on mailAPp. Please checkout the MailApp document Hope this helps.
Try this
Replace the MailApp.setEmail code with Following code
MailApp.sendEmail(email, subject, message, {replyTo:"youremail@domain.com"});
Hi I don't get this – the script emails me which isn't really a 'reply' is it? How do you get it to email the person who filled out the form?
Please make sure you have "Email" field in your contact form. This script is going to extract the email field from contact form. If its not provided then email will be sent to you.
I just updated the script with more clear error handling message. This should also make it more clear for others in future. Thanks for letting me know. Hope this will help you.
This is also updated in the post now. Thanks for feedback.
This is very helpful. Is there a way to get the email to include a field from a corresponding row in a different sheet?
@Ben – Thanks for comment. I am glad you found this useful.
I have not tried manipulating other sheet. What is the scenario you are trying to address? Let me know may be we can think of an alternative.
I got following error ;"Cannot read property "namedValues" from undefined."Please help me out
I have a questions before I can solve your problem.Have you created the contact form just like FromDev contact formPlease share you form link with me and I can verify that.
is it possible to change the from address so that it is different from the default option connected to your google account? I have all the forms set up on my personal google ID and I need the confirmation emails to go out from my work email ID. I do not want to have to migrate all existing forms and data to my work email ID.
I guess its best to transfer the ownership in that case. Its easy by going to the form – choose share then select the business email id to share the form with. By default it will give edit access, however you can choose is owner option in that setting. That way same forms will also be owned by business account. then you can follow the authorization steps inside business account to manage those forms.
It may be tedious if you have too many forms, but I do not see any other alternative as of now. Sending email with a differnt from address can be a security and spamming issue therefore it may not be allowed from google apps.
BTW I am assuming you business email is on google apps, otherwise you may not be able to share the ownership.
Let me know how it goes.
I got this question in email and thought of adding it to the comments since many others may also be looking for HTML emailsName :: Abdul KadirQuestion :: I read your article about "How-To Send Auto Reply Email on Submission of Google Docs Contact Form" in .And I glad it works for me. Previously, i'd like to turn my page with PHP mail() function for giving a feedback to users who fill the form, but looks like the method="POST" trigger is used by google on the form that I put on the same page. But the point why I use PHP, is because I can made the feedback email as HTML.Can I do it with the Google Script (Java Function) so i can make my available HTML "thanks page" appears on the feedback email? at least do you have an external link or maybe you already made that tutorial before?Really appreciate if you reply.
Abdul,Thanks for reaching out. You can easily send HTML format emails using google api. Replace this line MailApp.sendEmail(email, subject, message, {replyTo:myemail}); with this one MailApp.sendEmail(email, subject, message, {replyTo:myemail, htmlBody: "<html>Paste your HTML content here</html>'>"}); Hope this helps.For more details on this check the google document for this API –
can i embed another doc form into the auto reply email?
Shafeeque – I guess You mean to send a form inside the email. That can be easily done using the embed HTML code from google docs. Copy the html code into the email send function like this.
MailApp.sendEmail(email, subject, message, {replyTo:myemail, htmlBody:
"<html>Paste your HTML content here</html>'>"});
when i use the option for embed doc into website it gives an iframe code (<iframe src=……etc).. And while i put this iframe code into auto reply email as like u said, it shows an error in the script editor like this…..( line 38 missing } after property list)
Can you please post your code here? I can review and see what may be wrong.
HI, Not sure if my message was delivered. Everything is great and I appreciate the wisdom! My auto responder email is sending my message AND all of the info on the form. I only want the user to read my autoresponder message. How do I eliminate the info from the form submission.Thanks!!
Hello!This is great. I have a question. My autoresponse includes my message AND all of the info/answers on my form. How can I only have my response show?ThanksJC
Great Tip FromDev,Hope I can apply it to my site. Sorry to be a noob, but where can I find Tools – Script Editor? I looked at the Form maker page and it wasn't there.Thanks!
while i put iframe code into auto reply email as like u said, it shows an error in the script editor like this…..( line 38 missing } after property list). the code is gicen below.
iframe src="https://docs.google.com/spreadsheet/embeddedform?formkey=dFVVZ0plU3FQbDhaR1laaF9teFFGa2c6MA" width="760" height="659" frameborder="0" marginheight="0" marginwidth="0">Loading…</iframe
Try to replace all double quotes (") with single (') in this iframe snippet and see if that works.
It may look like this
src='https://docs.google.com/spreadsheet/embeddedform?formkey=dFVVZ0plU3FQbDhaR1laaF9teFFGa2c6MA' width='760' height='659' frameborder='0' marginheight='0' marginwidth='0'
Let me know if it still does not work.
Also here is what my Form looks like on Display:http://gyazo.com/9295b2866d453528aabff0662cdcca81
Hey I get 2 errors when I submit form:1. Failed to send email: no recipient2. Cannot read property "namedValues" from undefined.Here is a picture of the code:http://gyazo.com/d4abc0f8d97fd414224ca4dec1a17769Here is a picture of the contact list the code is assigned tohttp://gyazo.com/e020037d021e11fea17c9abb0e50defdHere is the code copied and pasted:function sendAutoReply(e){ var myemail="bigpoppa003@gmail.com"; var email=""; var subject="Re:"; var message="Hi ~Firstname~, "; try { for(var field in e.namedValues) { message +=field + ' :: ' + e.namedValues[field].toString(); if (field == 'Subject') { subject += e.namedValues[field].toString(); } if (field == 'Email') { email = e.namedValues[field].toString(); }}MailApp.sendEmail(email, subject, message, {replyTo:bigpoppa003@gmail.com}); } catch(e){ MailApp.sendEmail(myemail, "Error in Auto replying to contact form submission. No reply was sent.", e.message); }}In addition notice that in my message there is a Hey~FirstName~ . THis is because I was trying to get google to recognize the recipient's name. But I don't think that code would work. Do you know a way for that?
Hey I liked your tutorial and applied it but I keep getting 2 errors which are:1. Failed to send email: no recipient2. Cannot read property "namedValues" from undefinedThe code is as follows:function sendAutoReply(e){ var myemail="bigpoppa003@gmail.com"; var email=""; var subject="Re:Generating Free Leads"; var message="Hi ~Firstname~, Today, I'm going to share with you a free traffic generation system called LeadsLeap.com. If you have heard about this service and you have not registered your free account, I suggest that you do it now at http://www.leadsleap.com?referid=bigpoppa003 LeadsLeap is an ingenious system that combines the power of contextual advertising and network lead generation. In this system, you are allowed to post ads to 10 levels of network you have built. Unlike traditional network lead generation methods, LeadsLeap only allows members to post their ads in the form of contextual ad. This means your leads do not receive obtrusive ads from their uplines, which is a good thing because no one likes to receive junk ads. Moreover, LeadsLeap sends very informative and useful newsletters to their members. I've saved some of the newsletters for future reference. I'm sure you will benefit from the newsletter too. This is a free service. Register your account now at http://www.leadsleap.com?referid=bigpoppa003 Regards, Punith"; try { for(var field in e.namedValues) { message +=field + ' :: ' + e.namedValues[field].toString(); if (field == 'Subject') { subject += e.namedValues[field].toString(); } if (field == 'Email') { email = e.namedValues[field].toString(); }}MailApp.sendEmail(email, subject, message, {replyTo:bigpoppa003@gmail.com}); } catch(e){ MailApp.sendEmail(myemail, "Error in Auto replying to contact form submission. No reply was sent.", e.message); }}In addition the picture of my list is as follows:http://gyazo.com/e020037d021e11fea17c9abb0e50defdThe picture of the above code in the script is as follows:http://gyazo.com/d4abc0f8d97fd414224ca4dec1a17769Also, notice that I put ~FirstName~ in the message field. That is because I was trying to figure out if that would put the recipient's name in the ~FirstName~ part. But i dont think it would work. Do you know a way to do that?
Hi. Thanks for the tutorial. Do you know if there is anyway to pre-populate a google form with the recipients contact details, rather than have them enter them manually. I'd be lloking to obtain those contact details from another google spreadsheet.
Thanks for your help
Hi thanks vm for the tutorial. Do you know if there is anyway to pre-populate the form responses with the recipients contact details (name, email address etc) rather than have them enter them manually? This could be taken from another spreadsheet perhaps.
Thanks for your help
Thanks for your comments, let me try to help here. Please change the line
{replyTo:bigpoppa003@gmail.com}
to
{replyTo:myemail}
Replace the message text with a small test message and see if it works. If it does than I guess the message text need to be constructed based on max limit if not then make sure you use the exact form as we have in the tutorial.
I reviewed your form and it has only two fields. Subject and Message fields are missing in that form.
The ~FirstName~ will not work since its just a part of string. If you want to replace it with name of person than you may need to do some more coding in the form. e.g.
Step 1: add a First Name field in form.
Step 2: add a variable in your code to read first name field. Just like we are doing for Subject and Email.
Step 3: Construct the message with First name variable like this
"first part of message" + firstNameVariable + "second part of message";
It can be difficult. You could do it if every user accesses a separate link. Here is a simple trick you can use. Send each user a separate link with all pre-population details and when they use that link to fill up the form the fields will be already populated using simple trick described here –
Hi Sachin,Thanks so much for this great tutorial. My autoresponse includes my message AND all of the questions/answers on my form. How can I remove these fields and answers on the auto reply? I only want to send them a thank you note for filling out the form but i dont want to include their answers on the auto reply. Please help.Thanks,JCL
Argument too large: subject
how do i fix this
Please reduce the size of subject field. Try putting a small text in subject value. I would recommend using a drop down value so users can type. Or may be a max limit text field.
I do not have a subject field in my form it is a registration form for user to register for classes. i deleted the subject part from the code
my code
var myemail = "abc@youmail.com";
//Leave this field blank – it will be auto populated from "Email" field in
// your contact form. Make sure you have a "Email" Field in your contact form.
var email = "";
// This is going to be the subject of reply email
// You can change it as per your requirements
//var subject = "Re: ";
// The variable e holds all the form values in an array.
// Loop through the array and append values to the body.
var message = "Thanks for contacting FromDev.com. We will get in touch with you shortly. nn—–nn";
try {
for(var field in e.namedValues) {
message += field + ' :: '
+ e.namedValues[field].toString() + "nn";
if (field == 'Subject') {
subject += e.namedValues[field].toString();
}
if (field == 'entry.8.single') {
email = e.namedValues[field].toString();
}
}
// We are using Java MailApp util of Google Apps Script
// that sends the email.
MailApp.sendEmail(email, message, {replyTo:myemail});
} catch(e){
MailApp.sendEmail(myemail, "Error in Auto replying to contact form submission. No reply was sent.", e.message);
}
}
I have set up the for the same as you have suggested even though this is not quite what I am after just so I can figure out the coding. I am still getting an error message 'Cannot read property "namedValues" from undefine.'
My coding is
function sendAutoReply(e)
{
var myemail = "enlighten.life.coach.info@gmail.com";
var email = "";
var subject = "Re:Wellness Products and Community Projects";
// The variable e holds all the form values in an array.
// Loop through the array and append values to the body.
var message = "Thanks for contacting Enlighten Life Wellness Products. We will get in touch with you shortly. nn—–nn";
try {
for(var field in e.namedValues) {
message += field + ' :: '
+ e.namedValues[field].toString();
if (field == 'Subject') {
subject += e.namedValues[field].toString();
}
if (field == 'Email') {
email = e.namedValues[field].toString();
}
}
MailApp.sendEmail(email, subject, message, {replyTo:myemail});
} catch(e){
MailApp.sendEmail(myemail, "Error in Auto replying to contact form submission. No reply was sent.", e.message);
}
}
Your help is very much appreciated!
Make sure the function signature is like this
function sendAutoReply(e)
the variable e is passed by google API.
Natalee – Can you share the contact form with me please? I can check and let you know if there is something missing. The does not look wrong at a glance.
thats exactly how i have it and i still get that error i cant figure it out. there is no subject field
Not sure if you can view this link without logging in?Hope that works?
I just filled up the form and go a auto reply from you. It seems to be working. Have you tested it with your own account email just to see if that works out.
Is there any specific scenario where it does not work
——–Here is the reply I got——–
Thanks for contacting Enlighten Life Wellness Products. We will get in touch with you shortly.
—–
Name :: Sachin FromdevEmail :: software.wikipedia@gmail.comSubject :: Wellness ProductsMessage :: Natalee, This is Sachin, just doing a test on the form. Please ignore this message. Timestamp :: 11/20/2012 2:51:49
Can you please share you contact form with software.wikipedia at gmail.com
I will try to see if I can help you resolve this.
I sent you an email with the subject DualActionTactics. thanks for all the help!
I have a question….. if the Email column has a formula…. the app script doesn't work… cuz it gets the formula not value, so… How can i fix it?
Thanks in Advance….
Store the value of formula in a variable and pass it to the function.
Feel free to share your formula and I can review.
Hi,
Thank you for the script. Is it possible to Cc the form, I have a client who wants to receive a copy of the registrations. Not quite sure why as they can access the spreadsheet, but who am I to ask. Thanks
Thanks for the script I a have form which i would like to send a specific message base on the product a customer select.Such that when a customer select product A an auto reply with the price of the product will be send to customer base on the product selected
Hey! Thanks for the tutorial. But I don't want the people who had submmitted the entry to receive what they had entered in the form. What do I do?
how do you modify the information submitted in the form?
i'm looking at this section of your code and trying to figure out how to only include the responses to some of the questions, but not all.
// The variable e holds all the form values in an array.
// Loop through the array and append values to the body.
// var message = "Thanks for contacting FromDev.com. We will get in touch with you shortly. nn—–nn";
try {
for(var field in e.namedValues) {
message += field + ' :: '
+ e.namedValues[field].toString() + "nn";
if (field == 'Subject') {
subject += e.namedValues[field].toString();
}
if (field == 'entry.8.single') {
email = e.namedValues[field].toString();
}
}
I have to face same message Here is my code:function sendAutoReply(e){ // Remember to replace abc@youmail.com with your own email address // This will be used to send you a email notification in case of error on form // for example if someone entered a wrong email address. var myemail = "rauf.ahmed@iiu.edu.pk"; //Leave this field blank – it will be auto populated from "Email" field in // your contact form. Make sure you have a "Email" Field in your contact form. var email = ""; // This is going to be the subject of reply email // You can change it as per your requirements var subject = "Re: "; // The variable e holds all the form values in an array. // Loop through the array and append values to the body. var message = "Thanks for contacting FromDev.com. We will get in touch with you shortly. nn—–nn";try { for(var field in e.namedValues) { message += field + ' :: ' + e.namedValues[field].toString() + "nn"; if (field == 'Subject') { subject += e.namedValues[field].toString(); } if (field == 'Email') { email = e.namedValues[field].toString(); } } // We are using Java MailApp util of Google Apps Script // that sends the email. MailApp.sendEmail(email, subject, message, {replyTo:myemail});} catch(e){ MailApp.sendEmail(myemail, "Error in Auto replying to contact form submission. No reply was sent.", e.message); }}Here is the form:Thanks in anticipation
I have a question, I also want to embed a photo (a guest pass to my facility). I've tried html format by using img src and i've tried to also do my own scripting
with no result. the best i can do is provide a link to the image? Any thoughts on this? Sorry, I'm new to this whole thing!
The for loop to get a variable dump was invaluable to me to figure out how to pick out a specific variable (use the label name, not the actual variable name). Thank you! Google's pseudo programming is quite frustrating when you're used to just programming it for yourself…
BTW, I almost skipped over this tutorial because you called it Java. It's *not* Java. It's Javascript. They're not at all the same. 😉
Thanks you
I need the same thing. Did you figure it out?
Comment (//) line 24 and 25 in this code and that should remove the fields from message. Sorry for the late reply. Hope this helps others.
Some asked me about changing the content in auto reply so wanted to add it in this post :
You should be able to reply with any content you want using same script. Just make the variable with a new content. Make sure you use javascript string contact operator if text is too large.
for example
var message = ' Some text you want to send ' + ' Some more text ' + ' even more text';
this should send the message with very large text too. Most of the time problem may happen if you try to construct a string in one shot. Instead try the above approach by using plus operator and stick to around 40 characters in a string.
Hope this helps.
Regards,
Sachin
Sorry for late reply, It can be done in same way we are checking for each field. Just add a if condition check for MailApp.send method and send different emails for different conditions.
Sachin,Thank you for this script how it does not work: I keep getting an error: "Failed to send email: No Recipient"I've isolated the cause, but I'm not seeing why it's a problem. The script is not looping through the fields. When I debug I get a error reporting that 'e' is undefined and so is 'field'Here's the script:function sendAutoReply(e){ // Remember to replace abc@youmail.com with your own email address // This will be used to send you a email notification in case of error on form // for example if someone entered a wrong email address. var myemail = "terry@iproduceleads.com"; //Leave this field blank – it will be auto populated from "Email" field in // your contact form. Make sure you have a "Email" Field in your contact form. var email = ""; // This is going to be the subject of reply email // You can change it as per your requirements var subject = "Re: "; // The variable e holds all the form values in an array. // Loop through the array and append values to the body. var message = "Thanks for contacting FromDev.com. We will get in touch with you shortly. nn—–nn"; try { for(var field in e.namedValues) { message += field + ' :: ' + e.namedValues[field].toString() + "nn"; if (field == 'Email') { email = e.namedValues[field].toString(); } //if (field == 'Subject') { // subject += e.namedValues[field].toString(); // } } // We are using Java MailApp util of Google Apps Script // that sends the email. MailApp.sendEmail(email,subject,message, {replyTo:myemail});} catch(e){ MailApp.sendEmail(myemail,email, e.message); }}here's the form: The script does work when I replace email = ""; with an actual email address. This tells me the script is not reading through the fields on the form.I only have two fields in the google docs spreadsheet. First Name and EmailAny thoughts?Cordially,TerryPS. Thank you for the script and willingness to help out.
just tried your form. didnt work :(. Please make sure the field names are case sensitive. The email field should be named 'Email' according to this code.
if (field == 'Email') {
email = e.namedValues[field].toString();
}
Let me know if you are able to resolve this. Otherwise we may need to try giving more authorization on the form to me.
Also noticed one more thing. The catch block should be like this.
catch(e){
MailApp.sendEmail(myemail, "Error in Auto replying to contact form submission. No reply was sent.", e.message);
}
This is required since you will get notified in case the fields are having some data issues. May be someone enters a garbage in email field and its not able to send email.
Hey,I keep getting the following error: Cannot read property "namedValues" from undefined.or: Failed to send email: no recipientI can pay you via paypal if you can help me fix this asaphere is my form: and here is my code:function sendAutoReply(e) { var myemail = "erikegelko@gmail.com"; var Email = ""; var subject = "Re:Test Email"; // The variable e holds all the form values in an array. // Loop through the array and append values to the body. var message = "Thanks for contacting Enlighten Life Wellness Products. We will get in touch with you shortly. nn—–nn"; try { for(var field in e.namedValues) { message += field + ' :: ' + e.namedValues[field].toString(); if (field == 'Subject') { subject += e.namedValues[field].toString(); } if (field == 'Email') { email = e.namedValues[field].toString(); } } MailApp.sendEmail(Email, subject, message, {replyTo:myemail}); } catch(e){ MailApp.sendEmail(myemail, "Error in Auto replying to contact form submission. No reply was sent.", e.message); } }
HI can you please check this form This is the script is usedfunction sendAutoReply(e){ // Remember to replace abc@youmail.com with your own email address // This will be used to send you a email notification in case of error on form // for example if someone entered a wrong email address. var myemail = "xxxx.aneesh@gmail.com"; //Leave this field blank – it will be auto populated from "Email" field in // your contact form. Make sure you have a "Email" Field in your contact form. var email = ""; // This is going to be the subject of reply email // You can change it as per your requirements var subject = "Re: "; // The variable e holds all the form values in an array. // Loop through the array and append values to the body. var message = " We will get in touch with you shortly. nn—–nn";try { for(var field in e.namedValues) { message += field + ' :: ' + e.namedValues[field].toString() + "nn"; if (field == 'Subject') { subject += e.namedValues[field].toString(); } if (field == 'Email') { email = e.namedValues[field].toString(); } } // We are using JavaScript MailApp util of Google Apps Script // that sends the email. MailApp.sendEmail(email, subject, message, {replyTo:myemail});} catch(e){ MailApp.sendEmail(myemail, "Error in Auto replying to contact form submission. No reply was sent.", e.message); }}
Thanks for your post. What would the script be if I had only 3 fields in the form:
Name
Email address
Message
I've tried changing the field names in your script, but keep getting messages like:
Error in Auto replying to contact form submission. No reply was sent.
"email" is not defined.
"subject" is not defined.
etc.
Do I add the script to the form or to the spreadsheet?
Thanks
Thanks! This has been very useful.
Is it possible to set the response email to send from a a different address- not a Google address?
Thanks everyone for sharing your experiences. I also want to tell my knowledge and experience. You can make fake email address and can tell anyone but twist is you can still receive all emails on your personal email address. make anonymous email
Hi
Simply and clear your script. Very useful:)
Is it possible to populate data from another sheet?
The main sheet (Form Responses) contain sensible data, that I do not want to accidentally share, therefor I created a second sheet. Moreover, I created a Unique code which I'd like to be inserted in the auto reply message.
Thanks for your help
Hi Sachin
Did you find out any solutions on Ben's issue (Aug 7,2012):
Is there a way to get the email to include a field from a corresponding row in a different sheet?
Thanks
@Radusavin – Checkout this thread on SO –
Please checkout the thread about reading cell from a spreadsheet into script –
Hi. My question may already be answered above, but I cant see it anywhere. Will this auto-reply e-mail include the form just filled out by the user.
Hi. This may be already answered, but I can`t find it anywhere.Will this auto-reply e-mail include the form just filled out by the user?
Hi. This may be already answered, but I can`t find it anywhere.Will this auto-reply e-mail include the form just filled out by the user?
Hi Dev,
Wanted to check how can I include basic formatting (like bold, underline, color, etc.) in the message variable so that the email can be well formatted.
Thanks for this post, made my life easier. 🙂
+Skjalg – Yes it does include the user form content at the bottom of the email. Try our contact form and you will see the script in action with a automated email reply.
Thanks! It really helped:)
Hi Sachin,
I am getting the same error:
google form auto response Cannot read property "namedValues" from undefined.
What do you suggest?
Thanks
Yes, it does include the form filled by user at the bottom of email.
Anshul, You should be able to do it using HTML tags. Try something like these
bold words
oops the html showed up in real bold 🙂 the syntax is going to be
<b>bold words</b>
First I hope that you're still monitoring this post for questions.
I've been looking for code for this functionality for a long time, but I too only get a response sent to me, not to the email for the sender. My code is below:
function sendAutoReply(e)
{
// Remember to replace abc@youmail.com with your own email address
// This will be used to send you a email notification in case of error on form
// for example if someone entered a wrong email address.
var myemail = "xxxxxx_xxxxx@gmail.com";
//Leave this field blank – it will be auto populated from "Email" field in
// your contact form. Make sure you have a "Email" Field in your contact form.
var email = "";
// This is going to be the subject of reply email
// You can change it as per your requirements
var subject = "Re: Your request for information";
// The variable e holds all the form values in an array.
// Loop through the array and append values to the body.
var message = "Please use acct.. nn—–nn";
try {
for(var field in e.namedValues) {
message += field + ' :: '
+ e.namedValues[field].toString() + "nn";
if (field == 'Subject') {
subject += e.namedValues[field].toString();
}
if (field == 'Email') {
email = e.namedValues[field].toString();
}
}
// We are using JavaScript MailApp util of Google Apps Script
// that sends the email.
MailApp.sendEmail(email, subject, message, {replyTo:myemail});
} catch(e){
MailApp.sendEmail(myemail, "Error in Auto replying to contact form submission. No reply was sent.", e.message);
}
}
I have tried it multiple different ways. I know I saw one of your responses above that a message section has to be included. Two things I have not made mine a required question, as I really do not want comments from this form. Does question have to be a required question to work? In practice I need neither the subject or message section for my purposes.
The only other thing I can think of: Is this restricted in the Google forms code to deny sending to an Explorer email address – hotmail, live, etc.?
Right now I have set up my site using the form response to send my client the info I need to send, but this is not the image that I want to portray. Please tell me why this is not working for me. I use Windows 8 and Chrome. I get the same error messages as others: "Cannot read property "namedValues" from undefined, or Failed to send email: no recipient."
I won't forget the help, believe me.
I have been trying to add replyTo to the code below but have been unsuccessful. I put a message about replying to different address in email body but need it to actually appear in the email address when a recipient clicks reply. This would allow the message to go to a different address instead of my gmail. Can anyone correct code below to show where and how to add replyTo?
Thanks!
MailApp.sendEmail(userEmail,
"CareLogic Helpdesk Ticket #" + lastRow,
"Thanks for submitting your Help Desk request. nnI'll start " +
"working on it as soon as possible. You are currently " +
"number " +
(numNew + 1) + " in the queue. nnJohn BarnesnDo not reply directly to this email. Reply to me@mydomain if needed.",
{name:"John Barnes"});
}
I would like to add a replyTo address but have been unsuccessful. Very new to coding. Can anyone rework code below to indicate syntax for replyTo? I am able to display reply to address in the body of the email but don't know how to make code stop using my gmail address when a recipient clicks reply.
MailApp.sendEmail(userEmail,
"CareLogic Helpdesk Ticket #" + lastRow,
"Thanks for submitting your Help Desk request. nnI'll start " +
"working on it as soon as possible. You are currently " +
"number " +
(numNew + 1) + " in the queue. nnJohn BarnesnDo not reply directly to this email. Reply to myemail@fmydomain if needed.",
{name:"John Barnes"});
}
Hi. How do i get content reply and Set auto send email ?
In case you are looking for sending an email from a account outside google apps or gmail, You may also want to look into the account verification step mentioned at this page – https://support.google.com/mail/answer/22370?hl=en
This will authorize your gmail or google apps account to send email as another email.
Though you can not send a email from any arbitrary account since its a security issue.
Eugenio,
Just tried the form in your comment and seems to be working fine. I got a automated reply. Not sure if its a issue on specific browser?
To remove these fields remove this line from your script
message += field + ' :: ' + e.namedValues[field].toString() + "nn";
App working great but on the auto reply that form submitter receives the populated fields are show randomly in email e.g. it does not follow the Form or Google Spreadsheet order (see example below)
So how do I get the email reply to submit as per form order?
Hope make sense!
[
Phone5,12
Medical Aid Number7,14
Member 2: Surname9
Member 1 Surname:2
Medical Aid6,13
Timestamp11/22/2013 7:40:26
Team Name1
NOK8,15
First Name3,10
]
Please share your form url, the sequence of params are passed by google API so we have little control over it. In case you want the exact order than put the "if" condition for each field you want to add then append it explicitly. Just like we are doing for "subject" field in this code.
Hi Sachin,
I am the one who submitted the comments yesterday as anonymous. I still really need help. I am hoping you are still watching this thread.
Hi Sachin,
I am desperate for help…. I am trying to provide an email response to a completer of the form that contains the summary of items submitted (there are 9 of them) and also provide the URL so that if they need to update the responses they can go back to that. Here is the code that I have so far – I am not getting an email back – it says – no recipient.
function sendAutoReply(e)
{
// Remember to replace abc@youmail.com with your own email address
// This will be used to send you a email notification in case of error on form
// for example if someone entered a wrong email address.
var myemail = "myemail@.com";
//Leave this field blank – it will be auto populated from "Email" field in
// your contact form. Make sure you have a "Email" Field in your contact form.
var email = "";
// This is going to be the subject of reply email
// You can change it as per your requirements
var subject = "Re: Submission of screening patient for Study";
// The variable e holds all the form values in an array.
// Loop through the array and append values to the body.
var message = "Thanks for submitting the screening information. Your responses are noted below nn—–nn"; "Click on the link below if you need to edit your answersnn";
try {
for(var field in e.namedValues) {
message += field + ' :: '
+ e.namedValues[field].toString() + "nn";
if (field == 'PI Name') {
PIName += e.namedValues[field].toString();
if (field == 'Email') {
email = e.namedValues[field].toString();
}
if (field == 'Pt Initials Or Screening Number') {
PtInitialsOrScreeningNumber += e.namedValues[field].toString();
}
if (field == 'Date Of Screening') {
DateOfScreening += e.namedValues[field].toString();
}
if (field == 'Tissue Type') {
TissueType += e.namedValues[field].toString();
}
if (field == 'Estimated Shipping Date Of Tumor Tissue') {
EstimatedShippingDateOfTumorTissue += e.namedValues[field].toString();
}
if (field == 'Expected Date Of Randomization') {
ExpectedDateOfRandomization += e.namedValues[field].toString();
}
if (field == 'Expected Date Of First Treatment') {
ExpectedDateOfFirstTreatment += e.namedValues[field].toString();
}
if (field == 'Comment') {
Comment += e.namedValues[field].toString();
}
}
}
// We are using JavaScript MailApp util of Google Apps Script
// that sends the email.
MailApp.sendEmail(email, message, {replyTo:myemail});
} catch(e){
MailApp.sendEmail(myemail, "Error in Auto replying to contact form submission. No reply was sent.", e.message);
}
}
@DWS – It seems the google drive has some issues on running old google docs scripts. Not sure which version you are on. Can you please share your google docs form with me. I can review. The field names should be exactly same. So email field should be named "Email" (case sensitive)
I have shared the form and response spreadsheet with you (via your email address) – I hope this works! Thanks again.
I previously posted code in regards to getting the form URL for editing – not sure if you need that or not – if so I can provide it again.
Hello again,
I've been playing around with this more and was able to make a few adjustments and have just the fields that are not blank show up.
I changed this from above-
// Only include form values that are not blank
for (var keys in columns) {
var key = columns[keys];
if (e.namedValues[key]) {
message += key + ' :: ' + e.namedValues[key] + "nn";
}
}
TO –
// Only include form fields that are not blank
for ( var keys in columns ) {
var key = columns[keys];
if ( e.namedValues[key] && (e.namedValues[key] != "") ) {
message += key + ' – '+ e.namedValues[key] + "n";
}
}
My next goal was to be able to use html. I changed the code you have above and it worked (I took off an extra '> ) But now none of the filled in fields from the spreadsheet are in the reply. It's like added that code made it completely ignore the fields. Not even timestamp – Is it because I want it all. Can I only have it one way or the other? I was looking for HTML reply AND display form fields that are not blank. I hope you can help me. Thanks again!
Thank you for putting this script out there and for the updates. I was pleased to see that only the fields that were not blank should show up in reply. However, this is not happening for me. All the fields are showing up with blanks after them. I'm not sure if this is happening only to me? I did not modify the code, just copy and paste. Thanks again!
Thanks for this script. I've used the old script and it worked very well. On this new script, the reply is not including ALL the filled in columns. I was pleased to see that only keys that were filled in should show up but that is not happening for me. I've tested it a few times, I know I filled in data and can see it on the spreadsheet but still it is not all included in the reply. Is this only happening to me?? I wonder why? I have not modified you script at all – just copy & paste. Again, thank you for your service to all of us.
Can you help me with the last part of your comment? I'd like to create a unique code and have it be inserted in the auto reply. So far I can create a unique code using an arrayformula in the spreadsheet but it doesn't transfer to the email. Any help (beyond the excellent help I've already received from this post) would be appreciated
Any idea how to have this script also generate a unique ID and have that loaded into the spreadsheet as well as into the email sent to the customer?
I guess you can use the mysheet.getLastColumn() – this is the id of the column in the spreadsheet. May be prefix/suffix some some text if you like. But this will be unique since every entry goes in a new line on spreadsheet.
Awesome, thanks for sharing.