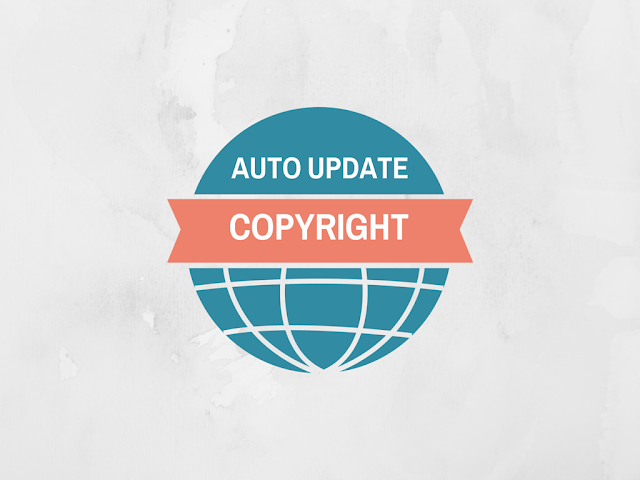
Recently I observed this with twitter.com when they forgot to update the Copyright year from 2009 to 2010 for few months. automatic copyright year update will avoid any such mistakes on your site. In this tutorial I am trying to capture most common ways where people may need to update copyright year in a website.
-
Using JSTL (JavaServer Pages Standard Tag Library)
-
Ant – Update at build time
-
PHP Application
-
JavaScript Copyright Year Update
-
WordPress
- By PHP
- By JavaScript
-
Blogger
JSTL provides rich tag support, we can use the core and format taglibs to format the year part of copyright message. Include below to taglibs in your JSP page.
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%> <%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt"%>
Now add the below code to the footer of your JSP page.
<c:set var="now" value="<%=new java.util.Date()%>" /> Copyright © <fmt:formatDate pattern="yyyy" value="${now}" /> My Company Name
This is a small ant target which can be used in your ant build script and can be used to update the application/company copyright year at build time. The below copyright.xml file extracts the (4 digit) year from system time-stamp and updates the property file that is storing copyright information (e.g. application.properties). copyright.xml
<project name="copyright" default="copyright"> <target name="init"> <property name="application.properties.file" value="application.properties"/> </target> <target name="copyright" depends="init"> <tstamp> <format property="copyright.year" pattern="yyyy"/> </tstamp> <echo>Copyright is: ${copyright.year}</echo> <propertyfile file="${application.properties.file}"> <entry key="app.copyright" value="Copyright © ${copyright.year} My Company Name"/> </propertyfile> </target> </project>
Content of application.properties before build script is run. application.properties
app.copyright=Copyright © 2009 My Copyright message
To see this sample running save the files (copyright.xml & application.properties) in same directory and run “ant -f copyright.xml” on command line in same directory. The copy right year should be updated in application.properties and the content of this file after running ant command should be something like this.
#Mon Apr 26 11:15:54 PDT 2010 app.copyright=Copyright © 2010 My Company Name
How to use this script
In case your application build.xml is too big and you don’t like to add new target in it then you can save the copyright.xml file as it is, and call it in your existing script as shown below
Make sure you update the application.properties file with appropriate file name and correct location. Ideally the update of copyright year should be done at run-time, therefore next few ways are for doing it during run-time using different web languages.
Updating Copyright year in a PHP based application can be really simple. Just add below code in your application footer section.
<p>Copyright © - <?php echo date('Y'); ?> My Company Name. All Rights Reserved.</p>
Below small JavaScript code snippet can be used to update copyright year in any web application. Just add below code in you footer section. (Thanks to Helen for suggesting this option in the comments.)
<p>Copyright © <script language="JavaScript" type="text/javascript">document.write((new Date()).getFullYear());</script> My Company Name</p>
In case you don’t want to use client side date object you can always initialize your JavaScript variable from a server side value.
There can be two ways to do this in WordPress
– Since WordPress uses PHP the same code can be used by WordPress blog owners to keep their blogs up to date with copyright year. Copyright message are usually added at the footer of the themes (most of times), so to add this code in your WordPress blog simply go to WordPress Dashboard and browse to the footer of the active theme and add this code then click Update. (If you are not a geek I suggest take a backup of your theme before doing any such change.)
<p>Copyright © - <?php echo date('Y'); ?> My Company Name. All Rights Reserved.</p>
Same JavaScript code can also be used in a WordPress theme to update the copyright year automatically. Just copy the below code and add in the WordPrese theme footer.
<p>Copyright © <script language="JavaScript" type="text/javascript">document.write((new Date()).getFullYear());</script> My Company Name</p>
Same JavaScript code can also be used in a Blogger templates to update the copyright year automatically. Just copy the below code and go to “Design” > “Edit Html”, and add the code below to the blogger template footer section. (If you are using blogger templates then search for [id=’footer-wrapper’])
<p>Copyright © <script language="JavaScript" type="text/javascript">document.write((new Date()).getFullYear());</script> My Company Name</p>
Please share your thoughts and suggestions in case you know a better way of doing the same thing.
Article Updates
- Updated on May 2019: Minor changes and updates to the introduction section. Images are updated to HTTPS.
Nice post. Your solution seem to work for those with apps based on Java. However, the same solution could achieved simply with JavaScript for those who don't have access to Java and Ant.Here's how it could be done: var currDate = new Date();document.write(currDate.getFullYear()); Inserting that into your copyright page ensures automatic update to the current year always.Nice post BTW 🙂Helen Neely
@Helen – Thanks for your comment. Using JavaScript is good idea. Though its just the year part, what If you don't want to rely on client date to be correct. May be we can initialize currDate from server side date variable.
I will update the post with your suggestion and some more options.
Thanks again.
Simple but great post. Hey, I've got the idea of the several coding for several applications. One thing that confused me that the PHP code can be used in the .html page's, whereas you have mentioned that PHP based application like home.php / index.php. Am I right? Please let me know as I'm a newbie. Thank you for your kind help.
Automatic Update of Copyright Year would be a great thing if it's possible, there are always a cases where one can forgot to update the copyright year, in such cases these code will prove useful where there will be an end to this problem for ever.
Thanks very much for this helpful post, it works great with the javascript code embedded in static html page. Great post indeed!!
Hi, Thanks a lot. It works. @Sam, yes, you can post the code on the html page and the code will work. The website needs to be php based though.
Very useful tips and information, javascript method is simple, nice and easy to make it, thank you for the great post!
Thank-you!!! I've just checked at least 6 different posts, many with the PHP solution you mention above. Unfortunately that didn't seem to work within the theme options 'copyright options' text box specific to the WordPress Avada theme. Attempts to update the footer.php file itself did not yield this text either, so I suspect it lives somewhere else. The brilliant thing, is that your Javascript snippet suggestion worked perfectly within the template's theme options area. FABULOUS. Thank you so much for having as many options outlined here as this. One of them is bound to work for everyone who stumbles upon this great post. Thanks again.
Thank you!!
Regarding your note for using javascript: "In case you don't want to use client side date object you can always initialize your JavaScript variable from a server side value."
how would one do this??