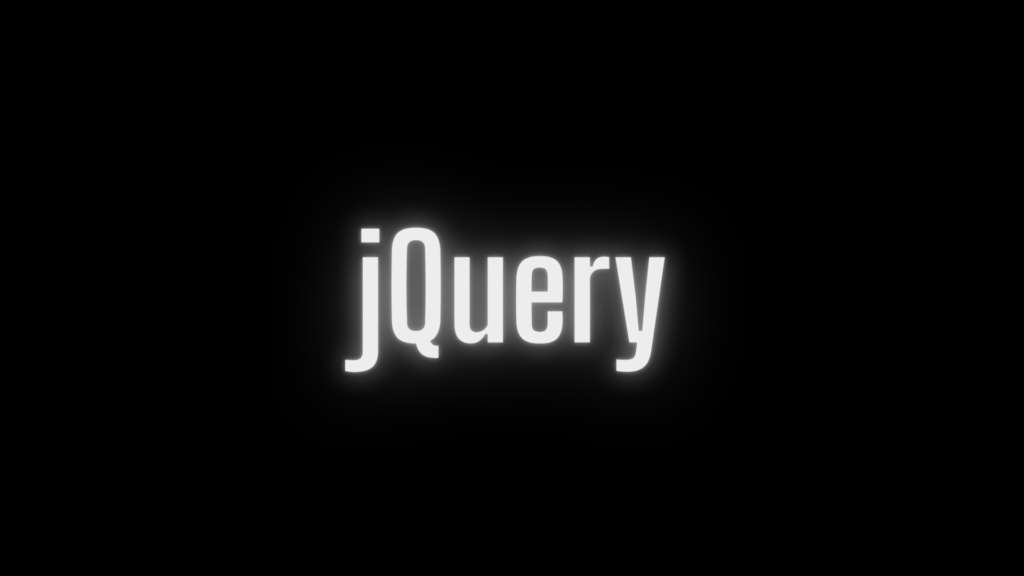
Manage Ajax Redirect Responses with jQuery: Complete Guide with Error Handling
In modern web applications, it’s common to perform actions asynchronously using jQuery Ajax — from login validation to form submissions and more. But what happens when, after completing an Ajax request, the server wants to redirect the user to another page?
Unlike traditional HTTP requests, Ajax doesn’t automatically follow HTTP redirect responses (like 302 or 301). This creates a unique challenge: developers need to explicitly manage redirection in the Ajax success handler.
This article provides a comprehensive, developer-friendly guide to managing redirect requests after a jQuery Ajax call. You’ll explore proven methods, complete with code examples and best practices, while also learning how to avoid common pitfalls.
Understanding Ajax and Redirects
What is Ajax?
Ajax (Asynchronous JavaScript and XML) is a technique that allows web pages to request data from a server asynchronously — without reloading the entire page. It improves performance and user experience in modern applications.
Why Redirects Don’t Work Normally with Ajax
In traditional requests, the browser automatically follows server-side redirects. However, in an Ajax call, the redirection response is received by JavaScript, not the browser. This means you must manually handle the redirection using JavaScript within the success or error callbacks.
Redirect Response Codes
- 302 Found: Temporarily redirects the client to another URL.
- 301 Moved Permanently: Indicates the resource has permanently moved.
- Location Header: Specifies the target URL for redirection in the HTTP response.
Browsers will follow these headers on full-page requests, but not inside Ajax responses.
Methods for Handling Redirects After Ajax
Let’s explore four robust methods to handle redirects after Ajax calls using jQuery.
1. Server-Side Redirect with Custom Header
How It Works
Instead of using a standard 302 redirect, the server sends a custom header (e.g., X-Redirect-Location
) indicating the redirect URL.
Example: PHP Server Response
header('Content-Type: application/json');
header('X-Redirect-Location: /dashboard.php');
http_response_code(200);
echo json_encode(["status" => "redirect"]);
Example: jQuery Ajax Handler
$.ajax({
url: '/login.php',
method: 'POST',
data: $('#loginForm').serialize(),
success: function(data, status, xhr) {
const redirectUrl = xhr.getResponseHeader('X-Redirect-Location');
if (redirectUrl) {
window.location.href = redirectUrl;
}
}
});
Pros and Cons
✅ Cleaner separation of logic
❌ Relies on custom headers (may require CORS config)
🔒 Security Tip: Validate the redirect URL server-side
2. JSON Response with Redirect URL
How It Works
The server sends a JSON object that includes the redirect URL.
Example: Node.js (Express)
res.json({ redirect: "/dashboard" });
Example: jQuery Ajax Handler
$.ajax({
url: '/login',
method: 'POST',
data: $('#loginForm').serialize(),
success: function(response) {
if (response.redirect) {
window.location.href = response.redirect;
}
}
});
Pros and Cons
✅ Simple to implement
✅ Easy to extend with other metadata
❌ Less standard for pure redirect semantics
🔒 Best Practice: Sanitize the URL before use
3. JavaScript Redirect in Success Handler
How It Works
Manually trigger a redirect using window.location.href
or window.location.replace()
after successful Ajax completion.
$.ajax({
url: '/submit-form',
method: 'POST',
data: $('#form').serialize(),
success: function(response) {
window.location.href = '/thank-you';
}
});
Or:
window.location.replace('/thank-you');
Pros and Cons
✅ Straightforward
✅ Full control over redirect logic
❌ Can mix business logic with UI logic
🔁 Use location.replace()
to avoid creating an extra entry in browser history
4. Dynamic Form Submission in Ajax Success
How It Works
Create a hidden HTML form dynamically and submit it, preserving the traditional form submission behavior.
$.ajax({
url: '/auth',
method: 'POST',
data: $('#loginForm').serialize(),
success: function(response) {
const form = $('<form>', {
action: '/dashboard',
method: 'POST'
});
$('<input>').attr({type: 'hidden', name: 'token', value: response.token}).appendTo(form);
form.appendTo('body').submit();
}
});
Use Case
Useful when the destination requires POST data and cannot be accessed via GET.
Error Handling and Edge Cases
Handling Ajax Errors
$.ajax({
url: '/process',
method: 'POST',
data: {},
success: function(response) {
if (response.redirect) {
window.location.href = response.redirect;
}
},
error: function(xhr, status, error) {
console.error('Request failed:', error);
alert('Something went wrong. Please try again.');
}
});
Common Edge Cases
- Invalid redirect URLs: Validate all server-side URLs.
- Network timeouts: Add fallback or retry logic.
- Session expiration: Handle 401/403 status codes properly.
SEO Considerations
Does Ajax Affect SEO?
Yes. Since Ajax does not trigger a full page load, search engines may not detect JavaScript-triggered redirects unless server-side rendering (SSR) or meta tags are used.
SEO Best Practices
- For content that should be indexed, prefer server-side 301/302 redirects.
- Use
meta refresh
or<noscript>
fallbacks for non-JavaScript users. - Consider server-side rendering frameworks for SEO-critical content.
Reference
Google SEO and JavaScript
Best Practices
- ✅ Always validate redirect URLs on the server to prevent open redirects.
- ✅ Use
window.location.replace()
for cleaner browser history. - ✅ Separate concerns: Keep business logic in the backend, UI logic in the frontend.
- ✅ Provide user feedback: e.g., loading spinners or success messages.
- ✅ Fail gracefully: Show errors if redirects fail or the server returns an error.
- ✅ Test on slow networks: Simulate low speeds to ensure redirects still function smoothly.
Troubleshooting Tips
- ❓ Not redirecting? Check if you’re inside a success handler.
- ❓ Getting CORS errors? Ensure custom headers are allowed in the server response.
- ❓ Multiple Ajax calls? Make sure the redirect only triggers once.
- 🔍 Use browser dev tools (Network tab) to inspect the server’s response.
Conclusion
Handling redirects after a jQuery Ajax call isn’t as simple as using Location:
headers — but with the right techniques, it’s manageable and secure.
We explored:
- Using custom headers and JSON to signal redirects.
- Executing client-side redirects with
window.location
. - Dynamically creating form submissions.
- Best practices and SEO implications.
Mastering these techniques ensures smooth, secure, and user-friendly redirection flows in your applications.
Did this article help you streamline your Ajax redirect workflow? Share it with your developer community or bookmark it for future use. Happy coding!