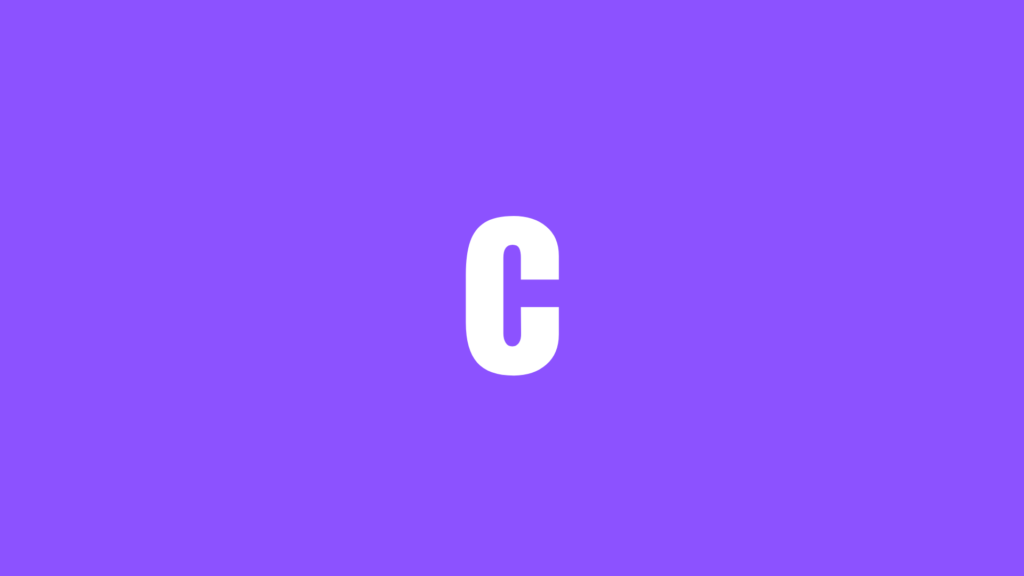
Ace Your C Programming: 10 Must-Know Questions That Separate Pros from Beginners
Are you confident in your C programming skills? Whether you’re preparing for a technical interview or simply want to test your knowledge, this comprehensive quiz will challenge your understanding of crucial C programming concepts. From memory management to pointer manipulation, these questions cover the fundamental aspects that every developer should master.
Why These Questions Matter
C remains a cornerstone of system programming, embedded systems, and high-performance computing. Understanding these core concepts isn’t just about passing interviews – it’s about becoming a more competent developer who can write efficient, reliable code. Let’s put your knowledge to the test!
The Quiz Challenge
Question 1: Pointer Arithmetic
cCopyint arr[] = {1, 2, 3, 4, 5};
int *ptr = arr + 2;
printf("%d\n", *ptr);
What will be the output of this code, and why?
Answer: The output will be 3
. When we add 2 to the array name, the pointer moves two integer positions forward (not bytes), pointing to the third element. This demonstrates how pointer arithmetic considers the size of the data type automatically.
Question 2: Memory Management
cCopychar *str = (char *)malloc(10);
strcpy(str, "Hello");
free(str);
str = NULL;
What potential issues should you watch out for in this code snippet?
Answer: While this code looks straightforward, there are several important considerations:
- Always check if malloc() returns NULL before using the allocated memory
- strcpy() doesn’t check buffer boundaries, making strncpy() a safer choice
- Setting str to NULL after free() is good practice but doesn’t prevent use-after-free bugs in other pointers to the same memory
Question 3: Structure Padding
cCopystruct Example {
char c;
int i;
char d;
};
printf("%lu\n", sizeof(struct Example));
What will be the size of this structure, and why isn’t it simply 6 bytes?
Answer: The size will typically be 12 bytes due to structure padding. The compiler adds padding bytes to ensure proper memory alignment for optimal CPU access. The actual layout might be:
- 1 byte for char c
- 3 bytes padding
- 4 bytes for int i
- 1 byte for char d
- 3 bytes padding
Question 4: Macro Magic
cCopy#define SQUARE(x) x * x
int result = SQUARE(3 + 2);
What will be the value of result? Is this macro implementation safe?
Answer: The result will be 13, not 25 as many might expect. The macro expands to 3 + 2 * 3 + 2
, which follows operator precedence rules. A safer implementation would be:
cCopy#define SQUARE(x) ((x) * (x))
Question 5: Static Variables
cCopyvoid counter() {
static int count = 0;
count++;
printf("%d\n", count);
}
What’s special about the behavior of this function when called multiple times?
Answer: The static variable count maintains its value between function calls. It’s initialized only once when the program starts, not each time the function is called. Each call will print incrementing numbers (1, 2, 3, etc.).
Question 6: Const Pointers
cCopyint value = 10;
const int *ptr1 = &value;
int *const ptr2 = &value;
What’s the difference between ptr1 and ptr2?
Answer:
- ptr1 is a pointer to a const int: The pointer can be changed to point to different locations, but you can’t modify the value it points to
- ptr2 is a const pointer to an int: The pointer must always point to the same location, but you can modify the value it points to
Question 7: Function Pointers
cCopyvoid (*fp)(int);
void print(int x) {
printf("%d\n", x);
}
fp = print;
What’s the purpose of function pointers, and how are they commonly used?
Answer: Function pointers allow for runtime polymorphism in C. They’re commonly used for:
- Callback mechanisms
- Plugin architectures
- State machine implementations
- Algorithm strategy patterns
Question 8: Volatile Keyword
cCopyvolatile int sensor_value;
while (sensor_value < threshold) {
// Do something
}
When and why should you use the volatile keyword?
Answer: Use volatile when dealing with:
- Hardware registers
- Variables accessed by interrupt service routines
- Variables shared between multiple threads It prevents compiler optimization that might cache the value in registers.
Question 9: Union Behavior
cCopyunion Data {
int i;
float f;
char str[4];
};
How does memory sharing work in unions, and what are the practical applications?
Answer: Unions share memory between all members, with the size being that of the largest member. Common uses include:
- Type punning (reinterpreting data)
- Memory optimization in embedded systems
- Implementing variant types
- Network protocol parsing
Question 10: Undefined Behavior
cCopyint a = 10;
a++ + ++a;
Why should you avoid such expressions, and what makes them undefined behavior?
Answer: This expression has undefined behavior because:
- The order of evaluations is not specified
- It modifies the same variable multiple times between sequence points
- Different compilers may produce different results Always write clear, unambiguous code that doesn’t rely on evaluation order.
Conclusion
How many questions did you get right? These concepts form the foundation of C programming expertise. Understanding them deeply will not only make you a better programmer but also help you write more reliable and efficient code.
Remember:
- Practice these concepts regularly
- Understand the “why” behind each behavior
- Write test programs to verify your understanding
- Review compiler documentation for implementation-specific details
Keep challenging yourself with similar questions, and don’t hesitate to dig deeper into the concepts that gave you trouble. The journey to C programming mastery is continuous, and every challenge is an opportunity to learn.
Further Learning Resources
To deepen your understanding of these concepts, consider:
- Reading the C standard documentation
- Experimenting with different compiler optimizations
- Contributing to open-source C projects
- Participating in coding challenges focused on C programming
Master these concepts, and you’ll be well-equipped to handle any C programming challenge that comes your way!